I want to center an unknown length of text (ok it's limited to a certain size, but I don't know if it needs one line or two lines) inside a div. Therfore I cannot use line-height
. I tried to use display: table-cell
, but than the layout is destroyed. I cannot use some tricks with top
because I need this for positioning.
Here is an example.
Mockup:
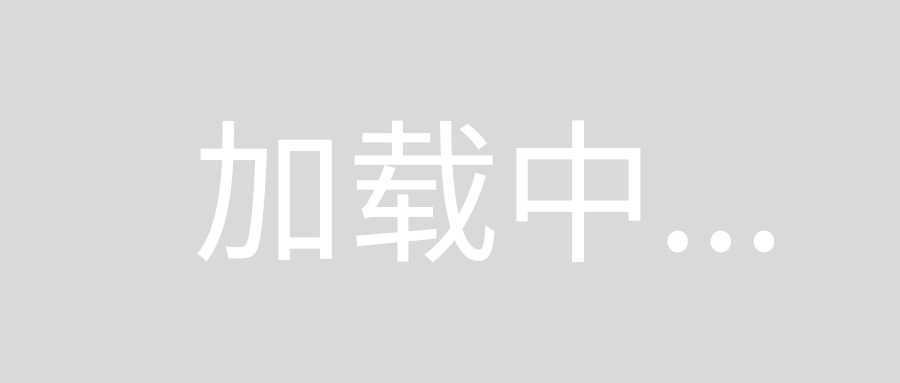
Original code:
HTML:
<div class="background"> </div>
<div class="TileText">MyText - Another long, long, long text</div>
CSS:
.background{
background-color:#000;
height: 400px;
width: 100%;
}
.TileText{
position: relative;
top: -135px;
max-width: 200px;
height: 80px;
background-color: #FFF;
color: #black;
clear: both;
padding-left: 10px;
}
Current state:
HTML:
<img src="images/castle.png" width="300" height="333" title="Castle" alt="Castle" />
<div class="TileTextWrapper">
<div class="TileText">Text</div>
</div>
CSS:
.TileTextWrapper{
position: relative;
top: -135px;
max-width: 200px;
height: 80px;
background-color: #FFF;
color: #57abe9;
clear: both;
padding-left: 10px;
display: table;
}
.TileText{
display: table-cell;
vertical-align: middle;
}
Update:
Now the text is vertically aligned. But I'm afraid display: table
would only work with modern browser.
I've updated your fiddle:
It seems to work
see: http://jsfiddle.net/hSCtq/11/ for reference.
I would put .TileText
inside of .background
. Creating an empty div for the sake of a background image is not semantically correct, and not necessary in most situations. One situation for a separate background div would be if you need to set the opacity of a background image separately from the content.
<div class="background">
<div class="TileText">MyText - Another long, long, long text</div>
</div>
.background {
background-color:#000;
height: 400px;
width: 100%;
display: table
}
.TileText{
max-width: 200px;
display: table-cell;
vertical-align: middle;
color: #fff;
clear: both;
padding-left: 10px;
}
Other questions with the same answer.
Horizontally and vertically center a pre tag without the use of tables?
The old center a image in a div issue ( image size variable - div size fixed )
Also, the first result from googling "center vertical text css" will give you the same code structure I posted in my JSfiddle
Add a <p>
tag inside your TileText div :
HTML
<div class="background"> </div>
<div class="TileText"><p>MyText - Another long, long, long text</p></div>
CSS
.background{
background-color:#000;
height: 400px;
width: 100%;
}
.TileText{
position: relative;
top: -135px;
max-width: 200px;
height: 80px;
background-color: #FFF;
color: #black;
clear: both;
padding-left: 10px;
}
.TileText p {
position:absolute;
display: table-cell;
vertical-align: middle
}
Example here: http://jsbin.com/ubixux/2/edit
You must add display:table-cell;
and also add vertical-align:middle;
To achieve vertical alignment, you have to use table. You can write your code as this:
<div style="width:600px; height:600px; border:#000000 1px solid;">
<table style="width:100%; height:100%; vertical-align:middle">
<tr>
<td>
MyText - Another long, long, long text
</td>
</tr>
</table>
</div>