I know that similar to this question was asked before, but mine is a little bit more different. I tried most of the previously suggested solutions, but none of them work.
So the problem is that I've got two classes as shown:
public class Dog
{
public String Name { get; set; }
public int Age{ get; set; }
}
public class Person
{
public String First_Name { get; set; }
public String Last_Name { get; set; }
public Dog dog { get;set;}
}
Also, I have a list of People List < Person > that I am displaying in datagridview.
The problem is when I display them I get something like
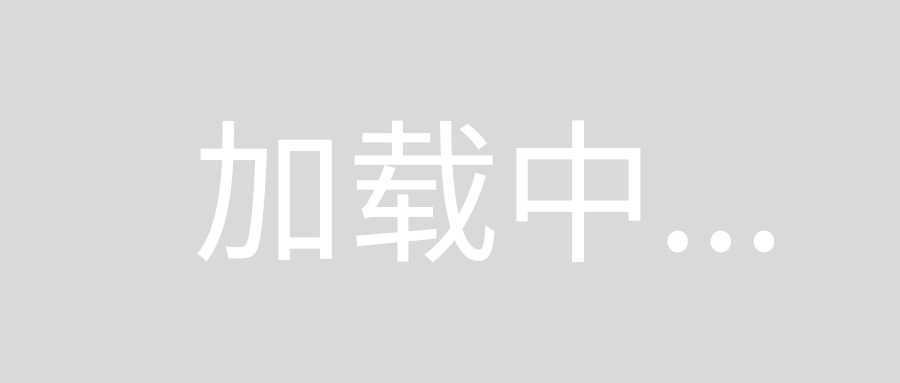
But what I need is:
The only way I managed to fix it is by returning only the Dog name in the To_String method, but it's not working for me because I need to return both of the name and the dog age.
I hope you understand me and can help me.
I'm surprised by the currently suggested (and even upvoted) answer that suggests creating another list just to change the display representation of a value.
What are you asking is called formatting and is supported by every UI framework/component. See my answer to changing the value of a cell in gridview at runtime for more details.
All you need is to handle CellFormatting event and put inside the handler something like this:
var dog = e.Value as Dog;
if (dog != null)
{
// Display whatever you like
e.Value = dog.Name;
e.FormattingApplied = true;
}
Full sample:
using System;
using System.Windows.Forms;
namespace Samples
{
static class Program
{
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
var form = new Form();
var dg = new DataGridView { Dock = DockStyle.Fill, Parent = form };
dg.CellFormatting += (sender, e) =>
{
var dog = e.Value as Dog;
if (dog != null) { e.Value = dog.Name; e.FormattingApplied = true; }
};
dg.DataSource = new[]
{
new Person { First_Name = "Ben", Last_Name = "Harison", dog = new Dog { Name = "Billy", Age = 50} },
new Person { First_Name = "Rob", Last_Name = "Jonson", dog = new Dog { Name = "Puppy", Age = 25} },
};
Application.Run(form);
}
}
public class Dog
{
public String Name { get; set; }
public int Age { get; set; }
}
public class Person
{
public String First_Name { get; set; }
public String Last_Name { get; set; }
public Dog dog { get; set; }
}
}
Result:
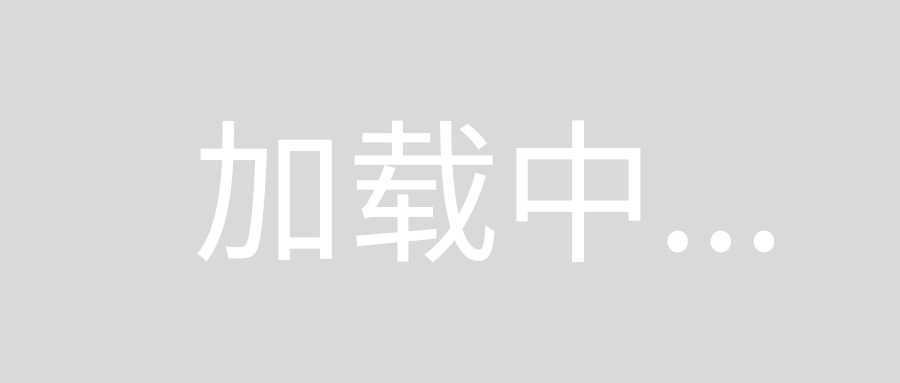
You can use Select function from LINQ:
this.datagridview.DataSource=youList.Select(x=>new {x.First_Name,x.Last_Name,Dog=x.Dog.Name}).ToList();