I have 4 divs in a container div. I want to be able to drag the contents of the four divs vertically but also be able to drag the container for all four divs horizontally. Something like the following..
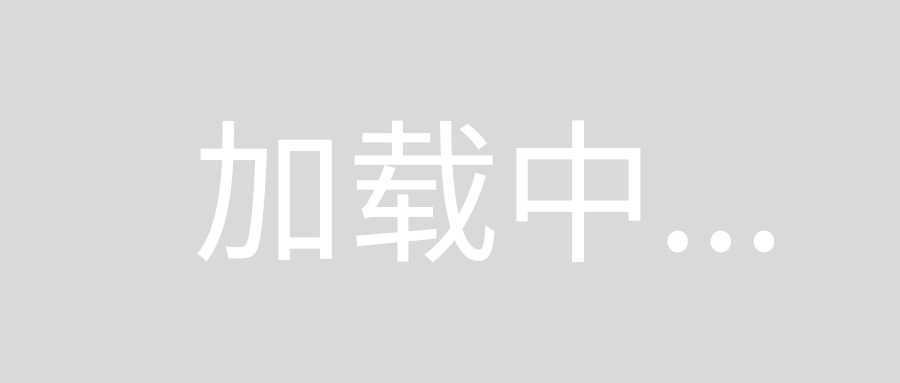
Or as seen here: http://jsfiddle.net/PuVCz/
Problem is you can drag the first two divs up and down when you click inside them and the whole lot left and right when you click inside the 2 right empty divs but you can't move the whole lot horizontally when you click in the first two as jquery thinks you are trying to control the up/down movement of the contents.
Is there a way of using draggable to achieve this?
PS Some code to please the Stack Overflow submit system but the pic or JSF show what I mean much better
$(function() {
$( "#content" ).draggable({axis: "x" });
$( ".fredcontent" ).draggable({axis: "y" });
});
I had the same problems..
my solution was to get in the drag state, the direction of movement.
start: function(e, ui) {
// get the start x coords to detect horizontal scroll
start = ui.position.left;
outerDragStartPos = parseInt(outerDrag.css('left'));
...
drag : function(event, ui){
// if horizontal scroll
if(ui.position.left != start){
if the direction was vertically, then nothing fascinating happens.
but if the script detects a movement in x axis then its disable "scroll x behaviour" and move the outer container instead.
// disable horizontal scroll for the internal container
innerDrag.draggable( "option", "axis", "y" );
// moving delay
if(ui.position.left > 30 || ui.position.left < -30){
outerDrag.css('left',outerDragStartPos + ui.position.left+"px");
i made a fiddle to show how it looks like.
I hope it helps a bit. ^‿^
Here is a working fiddle for you. The trick was to show some of the #content space so you can drag around it horizontally. Also added
.fredcontent{
height:100%;
}
so that you could drag the empty divs horizontally. Previously they did not have 100% height, hence the left and right dragging of the two empty divs was of
$( "#content" ).draggable({axis: "x" });
and not of
$( ".fredcontent" ).draggable({axis: "y" });
I have created a way to do this. Basically you apply drag and drop to all the child elements and if you drag across the X axis, it'll change the parent's X coordinates. Here it is on Fiddle.
Just add this to your parent element
$('#parent').doubleDragOnXY();
First it applies the .draggable() function to each of the child elements belonging to #parent
var children = $(this).children();
children.draggable({..})
Then in the drag property it tests to see if you are dragging it across the X axis or the Y axis. If you drag across the X axis, set the parents "left" css attribute to the new position. If you drag across the Y axis, return the new position:
if (xMax) {
var newLeft = parentStart + newPosition.left;
$(this).parent().css({
left: newLeft
});
newPosition.top = originalPosition.top;
newPosition.left = originalPosition.left;
}
if (!xMax) {
newPosition.left = originalPosition.left;
}
return newPosition;
Check it out on this fiddle.
http://jsfiddle.net/Dtwigs/mRPuv/
p.s. this code is heavily inspired by another example i found on stack overflow though I can't find/remember the example. basically it was allowing one object to move either on the or the Y but not both at the same time.