I have an authentication implemented via redirect to facebook auth page $fb->getLoginUrl()
and when this flow ends I'm getting a user access token.
According to the https://developers.facebook.com/docs/facebook-login/access-tokens/#extending it should be a short living one, while mine has the expiration in 2 months after today.
Is it an expected behaviour?
If yes - in what cases would you exchange the token?
If no - what am I missing?
UPD
I've just created a new application and again - the new user access token expires 1395567887 (in about 2 months)
(info from a token debugger)
Depends on what use case scenario you are implementing.
If you are using an APP and the token is for the app permissions (like if you use the getLoginUrl
to auth an app) etc then:
App tokens do not expire.
If your app publishes on behalf of its users and requires an access token with no expiration time for the purpose of publishing,
you should use an App Access Token. An App Access Token is signed
using your app secret and will not expire; it will be invalidated if
you re-key/reset your application secret.
EDIT
All my apps have a 2month access token by default and that is what is stated in the FB docs
Access tokens on the web often have a lifetime of about two hours, but
will automatically be refreshed when required.
Like described here:
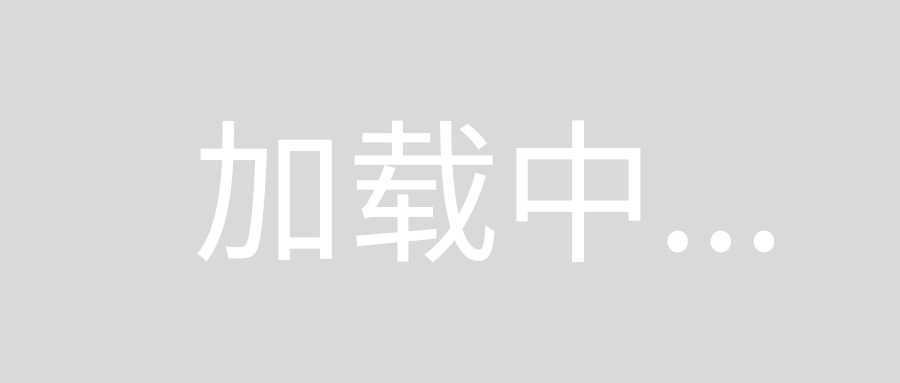
The server side flow grants a long lived token of 2 months
You can not get long lived access token by default, after authentication using facebook PHP-SDK.
You must have used / written following API function in your code block somewhere. Until and unless you make following API call, you won't be able to get long lived (2 month) token.
$facebook->setExtendedAccessToken();
You can also refer token Debugger: https://developers.facebook.com/tools/debug/accesstoken/
to check token details.
Edited
My code looks like as follows
$user = $facebook->getUser();
if ($user) {
try {
// Proceed knowing you have a logged in user who's authenticated.
$facebook->setExtendedAccessToken();
$user_profile = $facebook->api('/me');
} catch (FacebookApiException $e) {
error_log($e);
$user = null;
}
}
// Login or logout url will be needed depending on current user state.
if ($user) {
$logoutUrl = $facebook->getLogoutUrl();
} else {
$statusUrl = $facebook->getLoginStatusUrl();
$loginUrl = $facebook->getLoginUrl(array('scope' => 'read_stream, export_stream'));
}
If you can observe I have used setExtendedAccessToken if user details found after authentication. setExtendedAccessToken is a call which exchanges the temporary token with Long lived token.