I'm building a Sql query builder and would like to change the text color of a word in a textarea when the user types in words like SELECT, FROM, WHERE.
I've searched around a bit and beyond this (https://jsfiddle.net/qcykvr8j/2/) I unfortunately do not come any further.
Example code
HTML:
<textarea name="query_field_one" id="query_field_one" onkeyup="checkName(this)"></textarea>
JS:
function checkName(el)
{
if (el.value == "SELECT" ||
el.value == "FROM" ||
el.value == "WHERE" ||
el.value == "LIKE" ||
el.value == "BETWEEN" ||
el.value == "NOT LIKE" ||
el.value == "FALSE" ||
el.value == "NULL" ||
el.value == "TRUE" ||
el.value == "NOT IN")
{
el.style.color='orange'
}
else {
el.style.color='#FFF'
}
}
JSFiddle:
https://jsfiddle.net/qcykvr8j/2/
But this example deletes the color when I type further.
What I want is this:
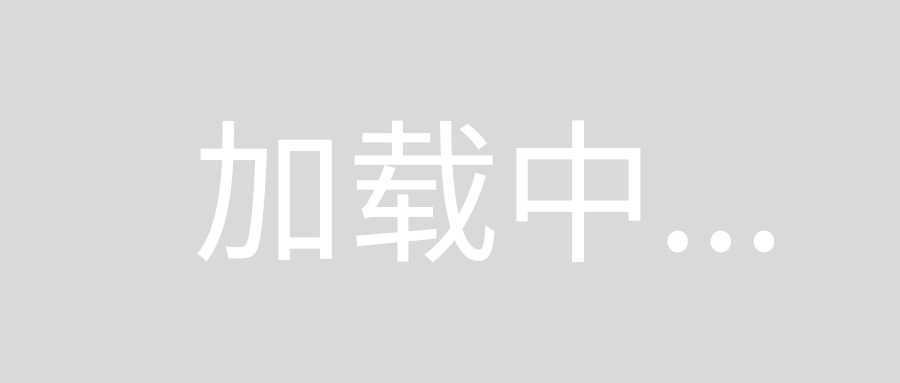
I've tried something with Keyup in combination with Contains in jQuery but that did not result in much.
Keyup: https://api.jquery.com/keyup/
Contains: https://api.jquery.com/contains-selector/
I hope someone can help me with an example or sites where I can find more information .
Regards, Jens
You can't change color words in textarea, but you can use contenteditable
attribute to change content text of element (like div, p, span). To do this work you can use javascript plugin, but if you want to create it, you can use this code. For this purpose, you need to get any word in text.Then check that if target word is in SQL statement highlight it.
$("#editor").on("keydown keyup", function(e){
if (e.keyCode == 32){
var text = $(this).text().replace(/[\s]+/g, " ").trim();
var word = text.split(" ");
var newHTML = "";
$.each(word, function(index, value){
switch(value.toUpperCase()){
case "SELECT":
case "FROM":
case "WHERE":
case "LIKE":
case "BETWEEN":
case "NOT LIKE":
case "FALSE":
case "NULL":
case "FROM":
case "TRUE":
case "NOT IN":
newHTML += "<span class='statement'>" + value + " </span>";
break;
default:
newHTML += "<span class='other'>" + value + " </span>";
}
});
$(this).html(newHTML);
//// Set cursor postion to end of text
var child = $(this).children();
var range = document.createRange();
var sel = window.getSelection();
range.setStart(child[child.length - 1], 1);
range.collapse(true);
sel.removeAllRanges();
sel.addRange(range);
$(this)[0].focus();
}
});
#editor {
width: 400px;
height: 100px;
padding: 10px;
background-color: #444;
color: white;
font-size: 14px;
font-family: monospace;
}
.statement {
color: orange;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id="editor" contenteditable="true"></div>