I have the need to display all values contained in a list in a table.
For example:
class Person {
String name;
String age;
List<Rule> rules;
}
the result that I expect:
|name |age |rules
alex 22 administrator, user, etc..
where administrator and user belong to Rule.name property
Does anyone know how to do that?
You should be able to add a generated column and format the values from the list as you need. You can find an example here, just scroll down to chapter 5.16.5 Generated Table Columns
Since you haven't posted any code, I don't know how you've setup your table. For convenience I have created a simple table with a BeanItemContainer<Person>
and I added a 'Rules' generated column. There's room for improvement but you should get the idea:
BeanItemContainer<Person> dataSource = new BeanItemContainer<Person>(Person.class);
table_1.setContainerDataSource(dataSource);
// create generated column and specify our "generator/formatter"
table_1.addGeneratedColumn("rules", new RuleGenerator());
dataSource.addAll(new ArrayList<Person>(){{add(new Person());}});
The formatter/generator:
public class RuleGenerator implements Table.ColumnGenerator {
@Override
public Object generateCell(Table source, Object itemId, Object columnId) {
Label label = new Label();
StringBuilder labelContent = new StringBuilder();
for(Rule rule : ((Person) itemId).getRules()){
labelContent.append(rule.getName()).append(",");
}
label.setValue(labelContent.toString());
return label;
}
}
This is what I got:
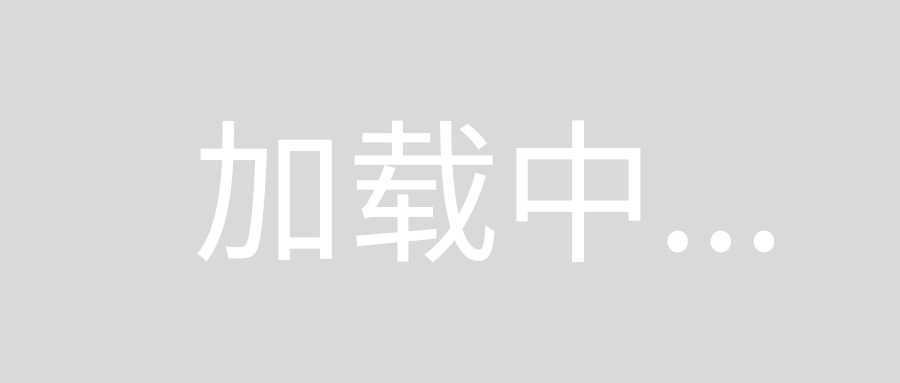
Are you using Vaadin 7? If yes, then use Converters. A converter is an interface that converts the value between datasource (in your case, collection) to presentation (in your case, string). There aren't any built-in collection <-> string converters in vaadin 7, but it is trivial to implement it yourself. Once you have implemented the converter, you can apply it on a column by calling table.setConverter(propertyId, converter);
The benefit of converters over column generators are 1) you can still sort/filter based on that column 2) you don't need to return a component, but simply a string. This creates less overhead and a lighter DOM structure that is faster for the browser to render.