我一直有在我建立一个应用程序这个问题。 请忽略所有的设计缺陷和缺乏最佳实践方法,这纯粹是为了展示什么,我解决不了的例子。
我有DialogFragment
它返回一个基本AlertDialog
使用自定义View
使用一套AlertDialog.Builder.setView()
如果此View
有特定的尺寸要求,我怎么得到Dialog
正确地调整自身以显示所有自定义内容的View
?
这是我一直在使用的示例代码:
package com.test.test;
import android.os.Bundle;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.DialogFragment;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.Paint.Style;
import android.view.Gravity;
import android.view.LayoutInflater;
import android.view.View;
import android.view.WindowManager;
import android.view.View.OnClickListener;
import android.view.ViewGroup;
import android.view.ViewGroup.LayoutParams;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.FrameLayout;
import android.widget.LinearLayout;
import android.widget.Spinner;
import android.widget.TextView;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Use a button for launching
Button b = new Button(this);
b.setText("Launch");
b.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// Launch the dialog
myDialog d = new myDialog();
d.show(getFragmentManager(), null);
}
});
setContentView(b);
}
public static class myDialog extends DialogFragment {
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
// Create the dialog
AlertDialog.Builder db = new AlertDialog.Builder(getActivity());
db.setTitle("Test Alert Dialog:");
db.setView(new myView(getActivity()));
return db.create();
}
protected class myView extends View {
Paint p = null;
public myView(Context ct) {
super(ct);
// Setup paint for the drawing
p = new Paint();
p.setColor(Color.MAGENTA);
p.setStyle(Style.STROKE);
p.setStrokeWidth(10);
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
setMeasuredDimension(800, 300);
}
@Override
protected void onDraw(Canvas canvas) {
// Draw a rectangle showing the bounds of the view
canvas.drawRect(0, 0, getMeasuredWidth(), getMeasuredHeight(), p);
}
}
}
}
一个Button
被创建,这将打开DialogFragment
的点击。 自定义View
( myView
)需要具有的800的宽度和高度300被正确的重写设置onMeasure()
此View
,绘制其测量界限洋红色进行调试。
800宽度比默认更宽Dialog
我的设备上的大小,但被削波,而不是正确地伸展。
我已经通过了以下解决方案看:
- DialogFragment.getDialog返回null
- 如何控制默认的警告对话框中的Android的宽度和高度?
- 警告对话框或自定义警告对话框的大小
我已经推导出以下两种编码方式:
- 获取
WindowManager.LayoutParams
中的Dialog
,并使用覆盖这些myDialog.getDialog().getWindow().get/setAttributes()
- 使用
setLayout(w, h)
通过方法myDialog.getDialog().getWindow().setLayout()
我曾尝试他们无处不在,我能想到的(覆盖onStart()
在onShowListener
,之后Dialog
中创建和显示等),一般都可以得到,如果这两种方法能够正常工作LayoutParams
都提供特定的值 。 但每当WRAP_CONTENT
提供,没有任何反应。
有什么建议?
编辑:
的情况截图: 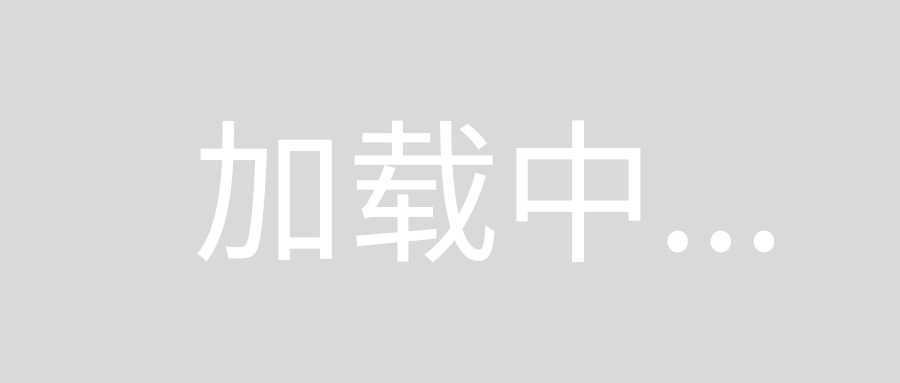
特定值的截图(注意900此输入,850不包括查看,这使得给整个窗口的意义正在调整的整个宽度,以便提供-如果另一个需要-之所以WRAP_CONTENT
是必不可少/固定值是不恰当的): 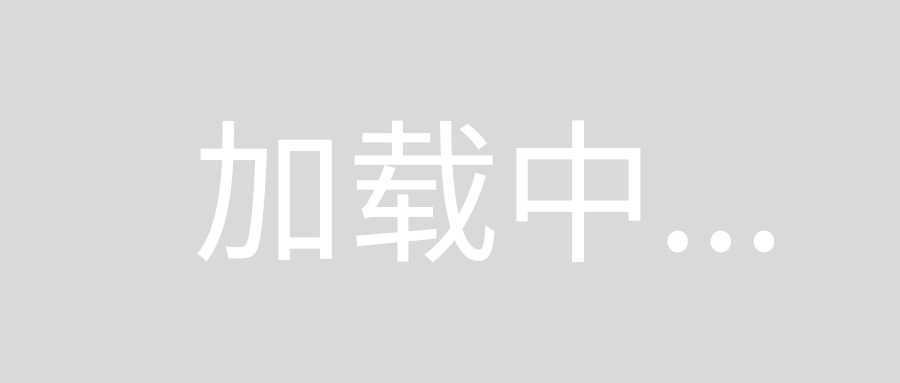
Answer 1:
我有一个工作的解决方案,说实话,我想办法挖掘过深,以获得这样一个简单的结果。 但在这里,它是:
究竟正在发生的事情:
通过打开Dialog
的布局层次浏览器 ,我可以检查整个布局AlertDialog
和究竟在发生什么: 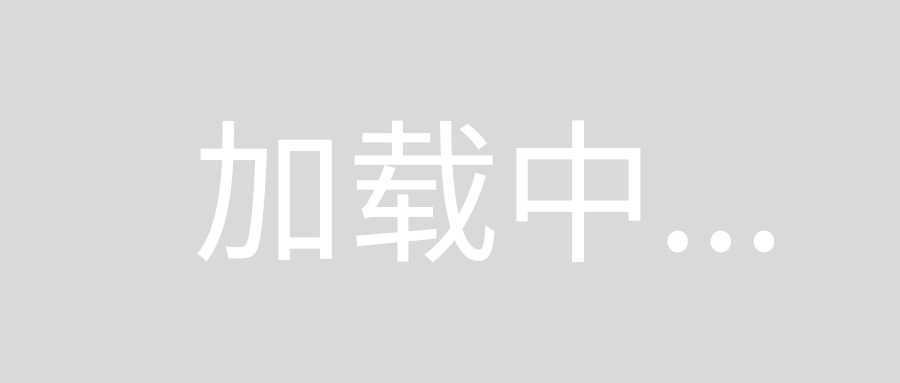
蓝色亮点是所有的高电平部分(的Window
,帧为Dialog
视觉样式等),并从蓝色向下的端部,其中用于所述部件AlertDialog
是( 红色 =标题, 黄色 =一个滚动视图存根,也许对于列表AlertDialog
S, 绿 = Dialog
内容即自定义视图, 橙 =按钮)。
从这里很明显的是,7收看路径(从蓝色到绿色的末尾开始)是什么无法正确WRAP_CONTENT
。 纵观LayoutParams.width
每个View
显示,所有被赋予LayoutParams.width = MATCH_PARENT
和地方(我想在顶部)大小设置。 所以,如果你遵循的树,很显然,您的自定义View
在树的底部,将永远无法影响的大小Dialog
。
那么,什么是做现有的解决方案?
- 这两个在我的问题中提到的编码方法简单地获得顶部
View
和修改它LayoutParams
。 显然,所有的View
相匹配的父母在树对象,如果顶层设置一个静态的大小,整个Dialog
会改变大小。 但是,如果顶层设置为WRAP_CONTENT
时,所有的休息View
树中的对象仍然仰视树 “匹配他们的父母”,而不是向下看树 “包装他们的内容”。
如何解决这个问题:
说白了,改LayoutParams.width
所有的View
中的影响路径对象是WRAP_CONTENT
。
我发现,这只能完成后onStart
的生命周期步DialogFragment
被调用。 因此, onStart
实现,如:
@Override
public void onStart() {
// This MUST be called first! Otherwise the view tweaking will not be present in the displayed Dialog (most likely overriden)
super.onStart();
forceWrapContent(myCustomView);
}
然后,该功能适当地修改View
层次LayoutParams
:
protected void forceWrapContent(View v) {
// Start with the provided view
View current = v;
// Travel up the tree until fail, modifying the LayoutParams
do {
// Get the parent
ViewParent parent = current.getParent();
// Check if the parent exists
if (parent != null) {
// Get the view
try {
current = (View) parent;
} catch (ClassCastException e) {
// This will happen when at the top view, it cannot be cast to a View
break;
}
// Modify the layout
current.getLayoutParams().width = LayoutParams.WRAP_CONTENT;
}
} while (current.getParent() != null);
// Request a layout to be re-done
current.requestLayout();
}
这里是工作的结果: 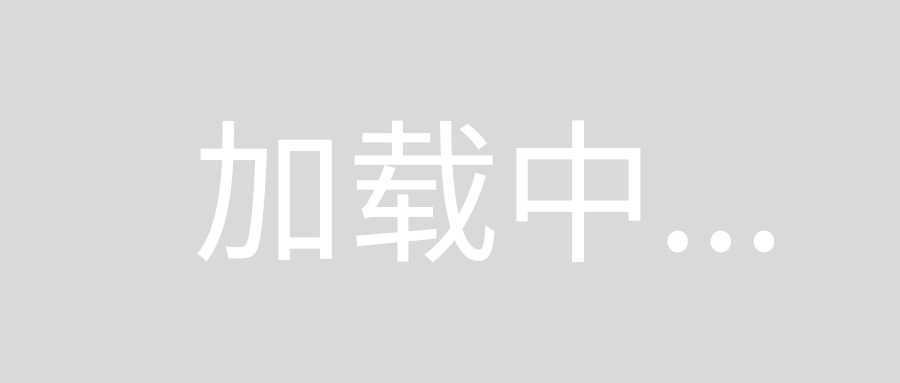
这让我困惑,为什么整个Dialog
不想被WRAP_CONTENT
有一个明确的minWidth
设置为处理所有符合预设的尺寸内的所有情况,但我敢肯定有它一个很好的理由事情是这样的(有兴趣听到)。
Answer 2:
后
dialog.show();
只是使用
dialog.getWindow().setLayout(ViewGroup.LayoutParams.WRAP_CONTENT, yourHeight);
很简单的解决方案,但它为我工作。 我延伸的对话,虽然,但假设这会为DialogFragment工作也。
Answer 3:
AlertDialog的使用这两个窗口属性来定义他们可以使平板电脑上的他们漂浮在屏幕中央的合理宽度的最小尺寸。
http://developer.android.com/reference/android/R.attr.html#windowMinWidthMajor http://developer.android.com/reference/android/R.attr.html#windowMinWidthMinor
您可以扩展您所选择的默认对话框样式和更改值应用自己的逻辑。
Answer 4:
这确定为我工作:
WindowManager.LayoutParams lp = new WindowManager.LayoutParams();
lp.copyFrom(dialog.getWindow().getAttributes());
lp.width = WindowManager.LayoutParams.WRAP_CONTENT;
lp.height = WindowManager.LayoutParams.WRAP_CONTENT;
dialog.show();
dialog.getWindow().setAttributes(lp);
Answer 5:
我发现一个问题BT的答案 。 对话已经离开神韵:(不,位于屏幕中心)。 为了解决这个问题,我添加改变父母的布局重心。 见更新forceWrapContent()方法。
protected void forceWrapContent(View v) {
// Start with the provided view
View current = v;
// Travel up the tree until fail, modifying the LayoutParams
do {
// Get the parent
ViewParent parent = current.getParent();
// Check if the parent exists
if (parent != null) {
// Get the view
try {
current = (View) parent;
ViewGroup.LayoutParams layoutParams = current.getLayoutParams();
if (layoutParams instanceof FrameLayout.LayoutParams) {
((FrameLayout.LayoutParams) layoutParams).
gravity = Gravity.CENTER_HORIZONTAL;
} else if (layoutParams instanceof WindowManager.LayoutParams) {
((WindowManager.LayoutParams) layoutParams).
gravity = Gravity.CENTER_HORIZONTAL;
}
} catch (ClassCastException e) {
// This will happen when at the top view, it cannot be cast to a View
break;
}
// Modify the layout
current.getLayoutParams().width = ViewGroup.LayoutParams.WRAP_CONTENT;
}
} while (current.getParent() != null);
// Request a layout to be re-done
current.requestLayout();
}
Answer 6:
对话应该是包裹内容
你可以用与透明背景和重心 match_parent的父布局 。 并把其下主布局。 所以它看起来像中心定位对话框。
通过这种方法,您可以使用滚动型,RecyclerView和对话的任何类型的布局。
public void showCustomDialog(Context context) {
Dialog dialog = new Dialog(context);
dialog.requestWindowFeature(Window.FEATURE_NO_TITLE);
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
view = inflater.inflate(R.layout.dialog_layout, null, false);
findByIds(view); /*find your views by ids*/
((Activity) context).getWindow().setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_STATE_VISIBLE | WindowManager.LayoutParams.SOFT_INPUT_ADJUST_RESIZE);
dialog.setContentView(view);
final Window window = dialog.getWindow();
window.setLayout(WindowManager.LayoutParams.MATCH_PARENT, WindowManager.LayoutParams.WRAP_CONTENT);
window.setBackgroundDrawableResource(R.color.colorTransparent);
window.setGravity(Gravity.CENTER);
dialog.show();
}
Answer 7:
使用setStyle(STYLE_NORMAL, android.R.style.Theme_Holo_Light_Dialog);
Answer 8:
只需使用AppCompatDialog
import android.content.Context;
import android.os.Bundle;
import android.support.v7.app.AppCompatDialog;
import android.view.Window;
import android.widget.ProgressBar;
public class ProgressDialogCompat extends AppCompatDialog {
public ProgressDialogCompat(Context context) {
super(context);
supportRequestWindowFeature(Window.FEATURE_NO_TITLE);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
Context context = getContext();
int padding = context.getResources().getDimensionPixelSize(R.dimen.x_medium);
ProgressBar progressBar = new ProgressBar(context);
progressBar.setPadding(padding, padding, padding, padding);
setContentView(progressBar);
setCancelable(false);
super.onCreate(savedInstanceState);
}
}
文章来源: AlertDialog with custom view: Resize to wrap the view's content