I have an assignment in which I have to allow a user to plot a graph using a quadratic equation.
I managed to draw the skeleton of the graph, and now I am trying to display the "control panel" for the user to input the values.
I have 4 files:
graph.java
panel.java
panelB.java
panelC.java
My problem is when I run the code it is displaying only the panel.java
even in the container where it should display the other two panels.
panel.java
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.*;
import java.awt.geom.*;
import javax.swing.JPanel;
public class panel extends JPanel {
public panel(){
this.setBackground(Color.yellow);
}
}
Can anyone please advise what changes I should do to fix this problem?
I have done some changes to the graph.java file:
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.Dimension;
import javax.swing.*;
public class GraphApplet extends JApplet{
public GraphApplet(){
raph p = new Graph();//graph
p.setPreferredSize(new Dimension(760,500));
conn.add(p,BorderLayout.CENTER);
}
And now all that is being displayed is the graph.
Regarding the other code, I also made some changes to the class names:
gnjk;.java
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.*;
import java.awt.geom.*;
import javax.swing.JPanel;
public class Graph extends JPanel {
public Graph(){
this.setBackground(Color.yellow);
}
public void paintComponent(Graphics p) {
super.paintComponent(p);
Graphics2D graph = (Graphics2D)p;
this.setBackground(Color.yellow);//set background color.
int x,y,y1,x1,a,b,p1x,p1y,p2x,p2y;
int xstart = 7;
int ystart = 1;
int xfinish = 3;
.......
bhfvhn.java
import javax.swing.*;
import java.awt.*;
import javax.swing.JPanel;
public class ControlsA extends JPanel{
public void init (Box g) {
a = Box.createVerticalBox();
a.add(new JLabel("Please enter the values below:"));
a.add(new JLabel("h"));
}
}
jknmk.java
import javax.swing.*;
import java.awt.Component;
import java.awt.Dimension;
public class ControlsB extends JPanel{
public void init (Box b) {
b = Box.createHorizontalBox();
b.add(new JLabel("a"));
JTextField f1 = new JTextField("0.0");
f1.setMaximumSize(new Dimension(100,30));
b.add(f1);
}
}
Here is an updated to my project:
jkl.java
import java.awt.BorderLayout;
import java.awt.Container;
public class GraphApplet extends JApplet{
public GraphApplet() {
public void init(){
SwingUtilities.invokeLater(new Runnable() {
public void run(){
Container conn = getContentPane();
conn.setLayout(new BorderLayout());
Graph z = new Graph();
conn.add(p,BorderLayout.CENTER);
fasfae a = new ControlsA(box1);
conn.add(a,BorderLayout.LINE_START);
adsfawef b = new ControlsB(box2);
conn.add(b,BorderLayout.PAGE_END);
}
});
}
}
/*Container conn = getContentPane();
conn.setLayout(new BorderLayout());
Graph p = new Graph();//graph
p.setPreferredSize(new Dimension(460,560));
conn.add(p,BorderLayout.CENTER);
Box a = new Box(BoxLayout.Y_AXIS);
a.setPreferredSize(new Dimension(50,50));
conn.add(a,BorderLayout.EAST);
Box b = new Box(BoxLayout.X_AXIS);
b.setPreferredSize(new Dimension(201,50));
conn.add(b,BorderLayout.SOUTH);*/
//this code is commented not to loose it
vtk.java
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.*;
import java.awt.geom.*;
import javax.swing.JPanel;
class Graph extends JPanel {
public Graph(){
this.setBackground(Color.yellow);
}
@Override
public Dimension getPreferredSize(){return (new Dimension(460,560)); }
public void paint(Graphics z) {
Graphics graph = (Graphics2D)z;
this.setBackground(Color.yellow).
int x,y,y1,x1,a,b,p1x,p1y,p2x,p2y;
//line co-ordinates
//the numbers represent the number of boxes on the graph
int xstart = 7;
int ystart = 1;
int x = 3;
int y = 9;
//other variables
int i = 0;
int i2 = 0;
int m = 0;
int n = 0;
int m2 = 0;
int n2 = 0;
int f2 = 0;
int g2 = 1;
//ranges
int f = 5;
int g = -5;
//change -ve num to +ve
int g3 = Math.abs(g);
int a1 = g3 + f;
int b1 = a1;
a = (Height);
f = (Width);
}
}
}
// 6 variables the user has to input
}
@Override
public Dimension getPreferredSize() {return (new Dimension(200,100));}
}
nllkl.java
@Override
public Dimension getPreferredSize(){return (new Dimension(201,50));}
}
Still no improvement. I cannot understand what is going on.
Please try to run this code now, tell me is this closer to what you wanted ? if so do let me know, so that I can delete my answer. Do watch the changes I had done :
- in your
PanelC
class, where instead of using init()
method, I
made the constructor.
- Inside your Graph class< i had removed all
setPreferredSizes(...)
calls, and instead I had overridden getPreferredSize()
for each
Class extending JPanel
. For this visit each Class Panel, PanelB and
PanelC.
- Lastly I had changed the Dimension values supplied to the
JPanel
,
which is to go to the LEFT/LINE_START
side, to something that can
be seen
- BEST CHANGE is the use of EDT - Event Dispatch Thread, seems like you
really not aware of Concurrency in Swing
import java.awt.*;
import java.awt.geom.*;
import javax.swing.*;
public class Graph extends JApplet{
public void init(){
SwingUtilities.invokeLater(new Runnable()
{
public void run()
{
Container conn = getContentPane();
conn.setLayout(new BorderLayout());
Panel p = new Panel();//graph
conn.add(p,BorderLayout.CENTER);
Box box1 = new Box(BoxLayout.Y_AXIS);
PanelB a = new PanelB(box1);//vertical
conn.add(a,BorderLayout.LINE_START);
Box box2 = new Box(BoxLayout.X_AXIS);
PanelC b = new PanelC(box2);//horizontal
conn.add(b,BorderLayout.PAGE_END);
}
});
}
}
class Panel extends JPanel {
public Panel(){
this.setBackground(Color.yellow);
}
@Override
public Dimension getPreferredSize()
{
return (new Dimension(460,560));
}
public void paintComponent(Graphics p) {
super.paintComponent(p);
Graphics2D graph = (Graphics2D)p;
Dimension appletSize = this.getSize();
int appletHeight = (int)(appletSize.height);
int appletWidth = appletSize.width;
this.setBackground(Color.yellow);//set background color.
int x,y,y1,x1,a,b,p1x,p1y,p2x,p2y;
//line co-ordinates
//the numbers represent the number of boxes on the graph
int xstart = 7;
int ystart = 1;
int xfinish = 3;
int yfinish = 9;
//other variables
int i = 0;
int i2 = 0;
int m = 0;
int n = 0;
int m2 = 0;
int n2 = 0;
int f2 = 0;
int g2 = 1;
//ranges
int f = 5;
int g = -5;
//change -ve num to +ve
int g3 = Math.abs(g);
int a1 = g3 + f;
int b1 = a1;
y1 = (appletHeight);
x1 = (appletWidth);
y = (appletHeight / 2);
x = (appletWidth / 2);
a = (appletWidth / a1);
b = (appletHeight / b1);
int d = (appletWidth / a1);
int e = (appletHeight / b1);
/**
to determine the
ammount of pixles there
is in each box of the
graph, both y-axis and
x-axis
*/
int xbox = x1 / 10;
int ybox = y1 / 10;
//line variables
//the xstart, ystart, etc represent the number of boxes
//top point of the line on the graph
p1x = xbox * xstart;//start x
p1y = ybox * ystart;//start y
//lowwer point of the line on the graph
p2x = xbox * xfinish;//finish x
p2y = ybox * yfinish;//finish y
//draw y-axis numbers
//(+ve)
while(f != 0){
String s = String.valueOf(f);
p.drawString(s,(x + 5),m + 13);
m = m + b;
f = f - 1;
}
//(-ve)
m2 = y;
while(f2 != g-1){
String u = String.valueOf(f2);
p.drawString(u,(x + 5),m2 - 3);
m2 = m2 + b;
f2 = f2 - 1;
}
//draw x-axis numbers.
//(-ve)
while(g != 0){
String t = String.valueOf(g);
p.drawString(t,n,y - 5);
n = n + a;
g = g + 1;
}
//(+ve)
n2 = x + a;
while(g2 != g3+1){
String vw = String.valueOf(g2);
p.drawString(vw,n2 -10,y - 5);
n2 = n2 + a;
g2 = g2 + 1;
}
BasicStroke aLine2 = new BasicStroke(1.0F,
BasicStroke.CAP_ROUND,BasicStroke.JOIN_ROUND);
graph.setStroke(aLine2);
//notch on numbers and grid lines
//left to right, top to bottom notches
int v2 = -5;
int v5 = 0;
while(i <= a1-1){
p.setColor(Color.lightGray);//lightgray line
p.drawLine(a,0,a,y1);//vertical lightgray
p.drawLine(0,b,x1,b);//horizontal lightgray
a = a + d;
b = b + e;
i = i + 1;
}
//notches
while(i2 <= a1){
p.setColor(Color.blue);//notch color
p.drawString("x",v2+2,y+3);//xaxis
p.drawString("x",x-4,v5+4);//yaxis
v5 = v5 + e;
v2 = v2 + d;
i2 = i2 + 1;
}
//draws the border of the graph
p.setColor(Color.black);
Rectangle2D.Float rect = new Rectangle2D.Float(0,0,x1,y1);
BasicStroke aLine = new BasicStroke(2.5F,
BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND);
graph.setStroke(aLine);
graph.draw(rect);
//draw cross
BasicStroke aLine3 = new BasicStroke(2.5F,
BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND);
graph.setStroke(aLine3);
p.drawLine(x,0,x,y1); //vertical line
p.drawLine(0,y,x1,y); //horizontal line
//display the value of graph width and graph height
String aw = String.valueOf(x1);
p.drawString("Graph Width = ", 50,90);
p.drawString(aw,150,90);
p.drawString("Graph Height = ", 50,110);
String ah = String.valueOf(y1);
p.drawString(ah,156,110);
//draw line on graph
BasicStroke aLine4 = new BasicStroke(1.5F,
BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND);
graph.setStroke(aLine4);
p.setColor(Color.red);
if(p1x <= x1 && p2x <= x1 && p1y <= y1 && p2y <= y1){
p.drawLine(p1x,p1y,p2x,p2y);
Color c = new Color(0,0,0);
p.setColor(c);
p.drawString("X", p1x-4,p1y+4);
p.drawString("X", p2x-4,p2y+4);
}
else{
p.setColor(Color.black);
p.drawRect(48,34,223,35);
p.setColor(Color.white);
p.fillRect(49,35,222,34);
p.setColor(Color.red);
p.drawString("Wrong co-ordinates!!!", 50,50);
p.drawString("Values exceede applet dimensions.", 50,65);
}
}
}
class PanelB extends JPanel{
public PanelB (Box a) {
a = Box.createVerticalBox();
a.add(new JLabel("Please enter the values below:"));
a.add(new JLabel("a"));
JTextField g1 = new JTextField("0.0");
g1.setMaximumSize(new Dimension(100,30));
a.add(g1);
a.add(new JLabel("b"));
JTextField g2 = new JTextField("0.0");
g2.setMaximumSize(new Dimension(100,30));
a.add(g2);
a.add(new JLabel("c"));
JTextField g3 = new JTextField("0.0");
g3.setMaximumSize(new Dimension(100,30));
a.add(g3);
a.add(new JLabel("d"));
JTextField g4 = new JTextField("0.0");
g4.setMaximumSize(new Dimension(100,30));
a.add(g4);
a.add(new JButton("Plot"));
a.add(new JButton("Refine"));
add(a);
}
@Override
public Dimension getPreferredSize()
{
return (new Dimension(200,100));
}
}
class PanelC extends JPanel{
public PanelC (Box b) {
b = Box.createHorizontalBox();
b.add(new JLabel("a"));
JTextField f1 = new JTextField("0.0");
f1.setMaximumSize(new Dimension(100,30));
b.add(f1);
b.add(new JLabel("b"));
JTextField f2 = new JTextField("0.0");
f2.setMaximumSize(new Dimension(100,30));
b.add(f2);
b.add(new JLabel("c"));
JTextField f3 = new JTextField("0.0");
f3.setMaximumSize(new Dimension(100,30));
b.add(f3);
b.add(new JLabel("d"));
JTextField f4 = new JTextField("0.0");
f4.setMaximumSize(new Dimension(100,30));
b.add(f4);
b.add(new JButton("Plot"));
b.add(new JButton("Refine"));
add(b);
}
@Override
public Dimension getPreferredSize()
{
return (new Dimension(201,50));
}
}
Here is the HTML file I used :
<html>
<p> This file launches the 'Graph' applet: Graph.class! </p>
<applet code= "Graph.class" height = 550 width = 1000>
No Java?!
</applet>
</html>
Here is the output I am getting :
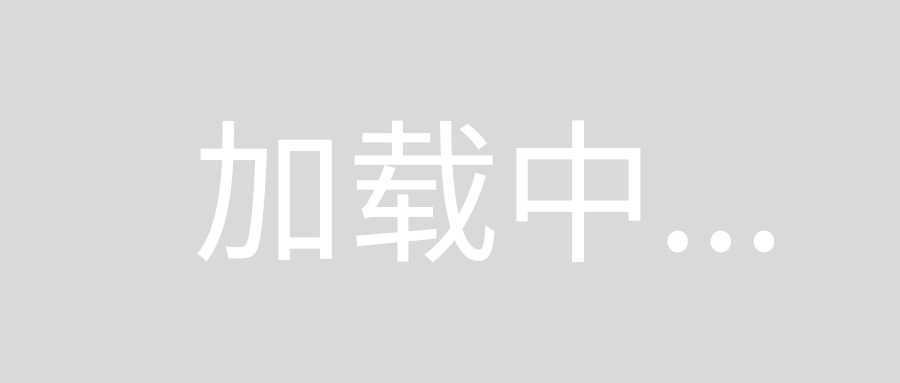
The main problems in that code are:
- The applet is adding 3 instances of
panel
as opposed to one each of panel
, panelB
& panelC
.
- Neither
panelB
& panelC
ever adds the Box
to the panel, so it will not appear.
- You intimate in the code that
panelB
should be vertically aligned, which means it would better fit in the LINE_START
(WEST
) of the BorderLayout
, as opposed to the NORTH
.
public void paint(Graphics p) {..
is wrong for panel
. Since panel
is a Swing JPanel
it should be public void paintComponent(Graphics p) {..
Once those things are attended to, this is how it might appear.
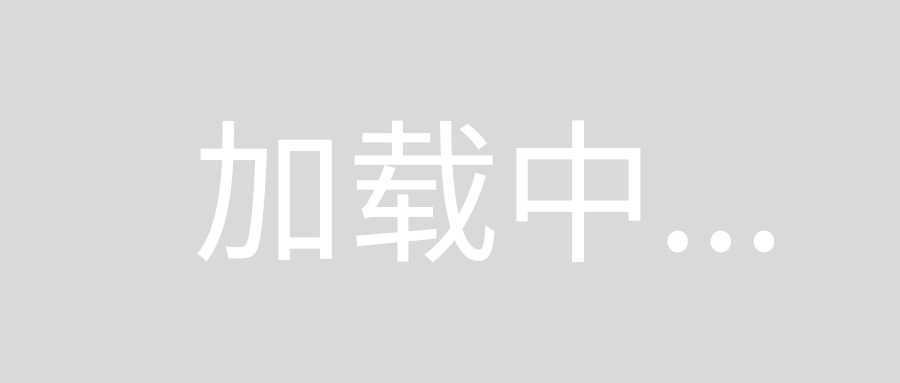
Other problems.
- The only code that needs to extend a class is for the applet itself and
panel
. In fact, even the panel
could be changed to show a BufferedImage
(the graph) inside a JLabel
- The
panelB
and panelC
are entirely redundant, just add a Box
directly to the layout area of the parent component needed.
- The nomenclature is wrong.
- Java class names should be
EachWordUpperCase
- Use meaningful class names - the applet might be
GraphApplet
, the graphing area Graph
, ..I am not sure what to call the last 2 panels, since they carry identical components. If there were but one, I might call it Controls
as a class (which is overkill for this), or controls
if it were an instance of a plain JPanel
or Box
.
- None of the calls to set maximum or preferred size are recommended in this situation. The only case that can be made for it is in the preferred size of the graph itself, but since this appears in the
CENTER
of applet, a size will be suggested by the applet width/height specified in HTML, minus the natural size of the other components (the CENTER
component will get 'the rest of the space').
Update
..how am I going to change the code in my applet so that the applet adds one of each panel(?)
Change:
panel a = new panel();//vertical
To:
panelB a = new panelB(new Box(BoxLayout.Y_AXIS));//vertical
Is the simplest way. Note that it changes if you decide to add the Box
directly.
Box a = new Box(BoxLayout.Y_AXIS);//vertical