I have a UIScrollView and there are many views inside my scroll view. I am using autolayout and all my views are layed out in the same manner: Left and top spacing to the superview, width and height set. Everything scrolls just fine, however my page control stays whereever it is. It does not scroll with the other elements inside the scroll view. YES, I did check that the page control is inside the scroll view just like the other elements, and yes, I've quadruple-checked the constraints of the page control. It just won't scroll. What could be the problem? There are labels, another scroll view, text views, images views and they all scroll perfectly, it's just the page view that is problematic. Is there a bug with Xcode/iOS SDK, or am I missing something?
UPDATE: All the views inside my scroll view are inside a container view. Both the scroll view's and the container view's translatesAutoresizingMaskIntoConstraints
property is set to NO
. It's only the page control that doesn't obey it's contraints. Here is a screenshot from the Interface Builder:
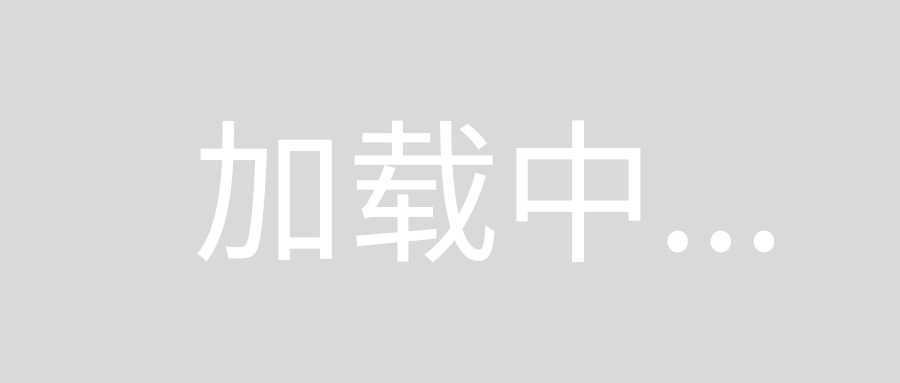
I got it to work by:
1) Putting all views/controls inside a containerView and declaring it as a property.
2) Adding this code:
-(void)viewDidLayoutSubviews{
[super viewDidLayoutSubviews];
self.scrollView.contentSize = self.containerView.bounds.size;
}
- (void)viewDidLoad{
[super viewDidLoad];
//added with containerview from g8productions
self.containerView = [[UIView alloc] initWithFrame:CGRectMake(36, 59, 900, 1200)];
self.scrollView.translatesAutoresizingMaskIntoConstraints = NO;
[self.scrollView addSubview:self.containerView];
[self.scrollView setContentSize:self.containerView.bounds.size];
}
Hope either of these solutions work for you!
I made something like that and I update the page control in the scrollview delegate methods:
-(void)scrollViewDidEndDecelerating:(UIScrollView *)scrollView
{
// If tag == 0 means that is the scrollview for gallery
if (scrollView.tag == 0)
{
self.pageControllGallery.currentPage = self.sv1ScrollViewGallery.contentOffset.x/320;
}
else
{
// Change the bottom label text
int page = (scrollView.contentOffset.x / 320);
[UIView animateWithDuration:0.3 animations:^{
self.labelBottom.alpha = 0.0f;
self.labelBottom.text = [self.arrayOfScrollviews objectAtIndex:page];
self.labelBottom.alpha = 1.0f;
}];
}
}
I calculate the page and set that page in PageControll.
Don't forget to set the Scrollview delegate in .h and in the element.
To size the scroll view’s frame with Auto Layout, constraints must either be explicit regarding the width and height of the scroll view, or the edges of the scroll view must be tied to views outside of its subtree.
https://developer.apple.com/library/ios/releasenotes/General/RN-iOSSDK-6_0/index.html
If you can dump Autolayout it would be best.
Check out that link, they've got a Pure AutoLayout example at the bottom.
Basically use this code pattern:
@interface ViewController () {
UIScrollView *scrollView;
UIImageView *imageView;
}
@end
@implementation ViewController
- (void)viewDidLoad{
[super viewDidLoad];
scrollView.translatesAutoresizingMaskIntoConstraints = NO;
imageView.translatesAutoresizingMaskIntoConstraints = NO;
[scrollView addSubview:imageView];
[self.view addSubview:scrollView];
NSDictionary *viewsDictionary = NSDictionaryOfVariableBindings(scrollView,imageView);
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|[scrollView]|" options:0 metrics: 0 views:viewsDictionary]];
[self.view addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|[scrollView]|" options:0 metrics: 0 views:viewsDictionary]];
[scrollView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"H:|[imageView]|" options:0 metrics: 0 views:viewsDictionary]];
[scrollView addConstraints:[NSLayoutConstraint constraintsWithVisualFormat:@"V:|[imageView]|" options:0 metrics: 0 views:viewsDictionary]];
}