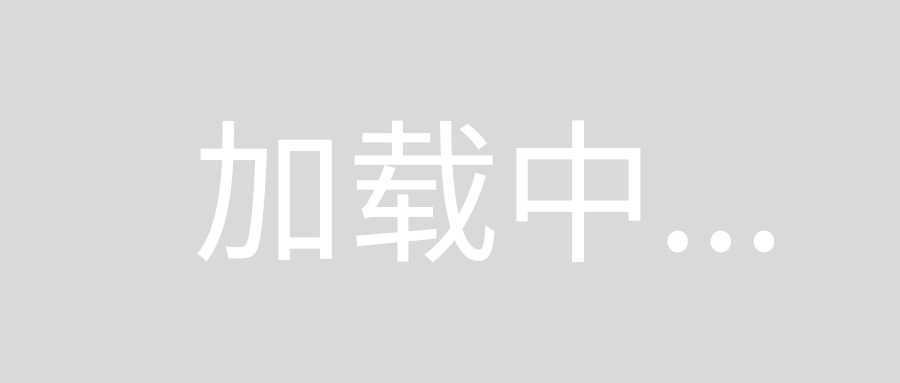
I am using angular google maps to display maps and markers. Is it possible to show the icon in the bottom right corner of the map that on click of it shows the current location.
<agm-map [latitude]="lat" [longitude]="lng" [zoom]="zoom">
<agm-marker *ngFor="let m of mapArrayList; let i = index"
[latitude]="m.geometry.location.lat()" [longitude]="m.geometry.location.lng()"
>
</agm-marker>
</agm-map>
First of all, Google Maps API v3 does not provide any default control for "show my location", so the only option would be implementing your own. But instead of creating it from scratch you could utilize a ready made control, e.g. klokantech.GeolocationControl
Here is an instruction on how to integrate it into Angular 2+ application using Angular Google Maps:
1)load maptilerlayer
library by putting it into index.html
:
<script src="https://cdn.klokantech.com/maptilerlayer/v1/index.js"></script>
or dynamically, for example using scriptjs
library:
get('https://cdn.klokantech.com/maptilerlayer/v1/index.js', () => {
});
2)initialize klokantech.GeolocationControl
in component:
app.component.html:
<agm-map #map [streetViewControl]=false [latitude]="lat" [longitude]="lng" [zoom]="zoom" (mapReady)="mapLoad($event)">
</agm-map>
app.component.js
export class AppComponent {
lat = -25.91;
lng = 145.81;
zoom = 4;
protected mapLoad(map) {
this.renderGeolocationControl(map);
}
renderGeolocationControl(map) {
get('https://cdn.klokantech.com/maptilerlayer/v1/index.js', () => {
const geoloccontrol = new klokantech.GeolocationControl(map, 18);
console.log(geoloccontrol);
});
}
}
Demo
Source code
Update
In case if you prefer to load https://cdn.klokantech.com/maptilerlayer/v1/index.js
dynamically, below is provided the instruction how to perform it via scriptjs
package:
Install the package:
npm i scriptjs
and type definitions for scriptjs
:
npm install --save @types/scriptjs
Then import $script.get()
method:
import { get } from 'scriptjs';
and finally load library:
get('https://cdn.klokantech.com/maptilerlayer/v1/index.js', () => {
const geoloccontrol = new klokantech.GeolocationControl(map, 18);
});