I have 200 products and I want to plot time vs parameter
graph. I have come up with a code that plots graphs for 20 products and displays them in one window.
I would like to know if there is a way to plot 200 graphs as subplots in 10 different windows, with each window containing 20 graphs each.
My Code
grouped = dataset.groupby('product_number')
ncols = 4
nrows = int(np.ceil(grouped.ngroups/40))
fig, axes = plt.subplots(figsize=(12,4), nrows = nrows, ncols = ncols)
for (key, ax) in zip(grouped.groups.keys(), axes.flatten()):
grouped.get_group(key).plot(x='TimeElapsed', y='StepID', ax=ax, sharex = True, sharey = True)
ax.set_title('product_number=%d'%key)
ax.legend()
plt.show()
This code gives me a window containing 20 subplots, as shown below
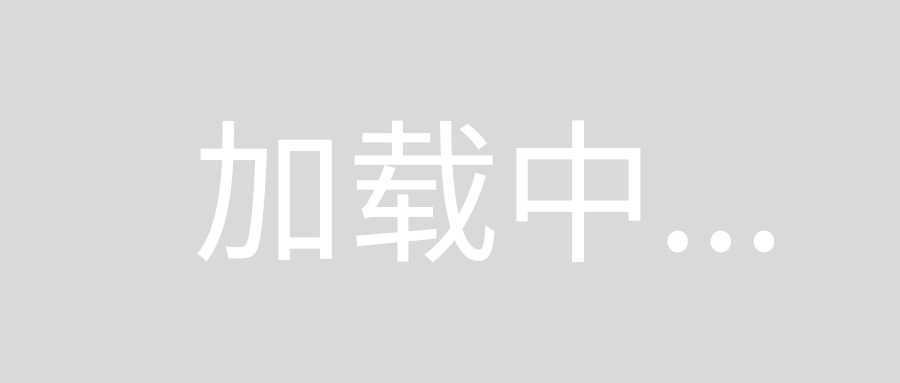
You just need to wrap your existing code in a for loop over different figures each containing 20 subfigures. The trick here is then to modify the key values using an index (20*i)+key
to get all 200 keys. For i=0
(first figure), you will get 1, 2, 3, ... 19, 20. For i=1
(second figure), you will get 21, 22, 23, ...39, 40 and so on.
Below is a modified version of your code. I do not have the data so I can't try it out. If it doesn't work, let me know. As pointed out by @DavidG, the plt.show()
should be outside the for loops.
grouped = dataset.groupby('product_number')
ncols = 4
nrows = int(np.ceil(grouped.ngroups/40))
for i in range(10):
fig, axes = plt.subplots(figsize=(12,4), nrows = nrows, ncols = ncols)
for (key, ax) in zip(grouped.groups.keys(), axes.flatten()):
grouped.get_group((20*i)+key).plot(x='TimeElapsed', y='StepID', ax=ax, sharex = True, sharey = True)
ax.set_title('product_number=%d'%((20*i)+key))
ax.legend()
plt.show() # Mind the indentation