Does it take into account altitude changes?
I mean, if I start in left vertex of this triangle and end in the right upper vertex, does it return distance a or b?
double distanceInMetersFloat = initialPosition.distanceTo(finalPosition);
In my app, both initialPosition and finalPosition are Location with altitudes (I set them with Google Elevation API).
According to Google:
Distance is defined using the WGS84 ellipsoid.
But you can do it with or without altitudes.
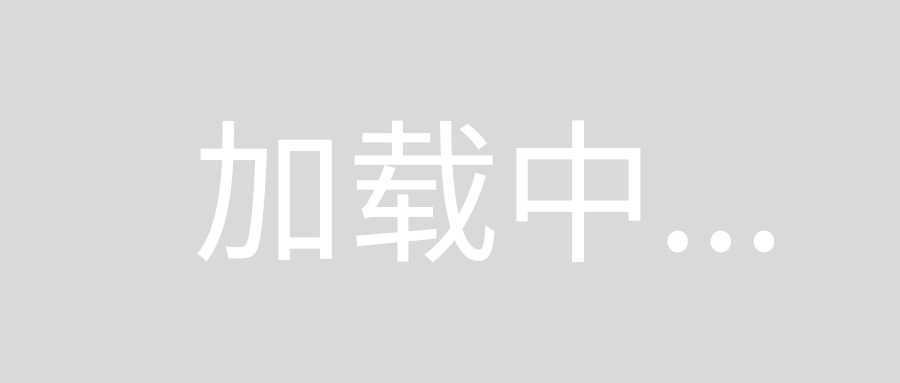
The altitude is not taken into account when computing Location.distanceTo
.
You can test it like this:
Location location1 = new Location("");
location1.setLatitude(40);
location1.setLongitude(-4);
location1.setAltitude(0);
Location location2 = new Location("");
location2.setLatitude(30);
location2.setLongitude(-3);
location2.setAltitude(0);
Location location3 = new Location("");
location3.setLatitude(30);
location3.setLongitude(-3);
location3.setAltitude(100);
Log.e("Without altitude", ""+location1.distanceTo(location2));
Log.e("With altitude", ""+location1.distanceTo(location3));
Log.e("Different altitude", ""+location2.distanceTo(location3));
This is the output:
E/Without altitude﹕ 1113141.5
E/With altitude﹕ 1113141.5
E/Different altitude﹕ 0.0