I'm trying to figure out how to make a custom EditText that has black borders along it's right and left sides, a green border on top, and a blue border on the bottom. See below:
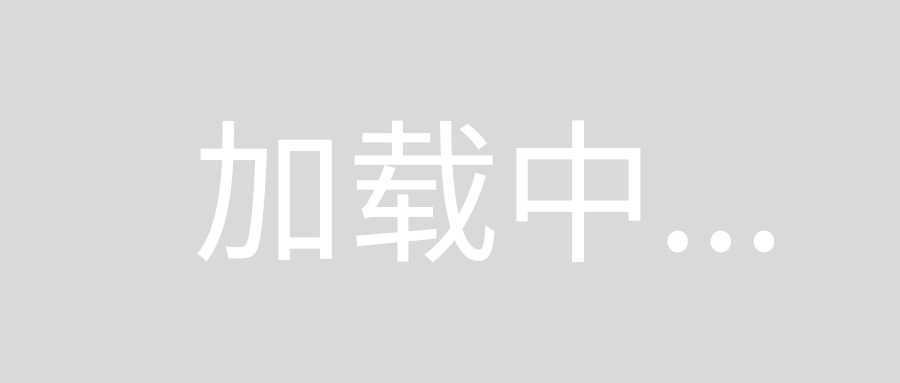
I'm fairly new to Android development and I've spent quite a lot of time reading their documentation but haven't had any luck finding anything on this kind of customization. I know in CSS you can just use the border-right, border-left, etc... properties but not sure if it's that straightforward in Android development. I'm looking for the solution that is the most compatible, preferably from version 2.3 (Gingerbread).
You'll have to make a custom image to use as the background. It's relatively straight forward, you'll want to use a 9-patch as described in the 2D graphics guide.
Once you have that, you'll put it in your res/drawable folder of your project, and then use it with the EditText in XML as
<EditText
android:background="@drawable/my_custom_background"
...
/>
create a LayerList
with a square colored in the wanted gradient, and above it a white square with some borders. then use this drawable as your TextView
background.
You can create a Multi-colored border EditText using 9-patch or in xml <layer-list>
here i am creating Multi-colored border EditText programmatically.
public class MainActivity extends AppCompatActivity {
private TextView mTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
customTextViewWithBorder();
}
private void customTextViewWithBorder(){
mTextView = (TextView) findViewById(R.id.tv);
// Initialize some new ColorDrawable objects
ColorDrawable leftBorder = new ColorDrawable(Color.RED);
ColorDrawable topBorder = new ColorDrawable(Color.GREEN);
ColorDrawable rightBorder = new ColorDrawable(Color.BLUE);
ColorDrawable bottomBorder = new ColorDrawable(Color.YELLOW);
ColorDrawable background = new ColorDrawable(Color.WHITE);
// Initialize an array of Drawable objects
Drawable[] layers = new Drawable[]{
leftBorder, // Red color
topBorder, // Green color
rightBorder, // Blue color
bottomBorder, // Yellow color
background // White background
};
// Initialize a new LayerDrawable
LayerDrawable layerDrawable = new LayerDrawable(layers);
// Red layer padding, draw left border
layerDrawable.setLayerInset(0,0,0,15,0);
// Green layer padding, draw top border
layerDrawable.setLayerInset(1,15,0,0,15);
// Blue layer padding, draw right border
layerDrawable.setLayerInset(2,15,15,0,0);
// Yellow layer padding, draw bottom border
layerDrawable.setLayerInset(3,15,15,15,0);
// White layer, draw the background
layerDrawable.setLayerInset(4,15,15,15,15);
mTextView.setBackground(layerDrawable);
// Set the TextView padding
mTextView.setPadding(25,25,25,25);
}
Source android--code