I'm attempting to generate an annotated LineChart using the google visualization API, and while I have it working, I would like to be able to have annotations have line-breaks if possible. Unfortunately, it seems like Google's API ignores any newline information and displays everything on a single line. Has anyone come up with a way around this?
Here's an example:
var data = new google.visualization.DataTable();
data.addColumn('string', 'Month');
data.addColumn('number', 'Sales');
data.addColumn({type:'string', role:'annotation'});
data.addColumn({type:'string', role:'annotationText'});
data.addRows([
['April',1000, 'A', "Stolen data\nSo-so month"],
['May', 1170, 'B', "Coffee spill\nAnother line\nA third line"],
['June', 660, 'C', "Wumpus attack"]
]);
I've tried \n, \\n, and <br />
and those aren't working.
Add p': {'html': true}}
to your tooltip like this
data.addColumn({type:'string', role:'tooltip','p': {'html': true}});
then your add <br/>
to your tooltip content
create a second annotation line.
var data = new google.visualization.DataTable();
data.addColumn('timeofday', 'Time');
data.addColumn('number', 'Temp');
data.addColumn({type:'string', role:'annotation'});
data.addColumn({type:'string', role:'annotation'});
data.addRows([
[[4, 56, 00], 0, '0', 'rain'], [[5, 56, 00], 10, '10', 'drizzle'], [[6, 56, 00], 23, '23', 'cats'], [[7, 56, 00], 17, '17', 'partly cloudy'], [[8, 56, 00], 18, '18', 'sunny'], [[9, 56, 00], 9, '9', 'sunny'], [[10, 56, 00], 11, '9', 'sunny'], ]);
Check this out https://jsfiddle.net/k6eocgLd/4/
I have implemented a simple example for you to implement multi-line annotation using Google Chart. Copy and paste the following example and run it.
<html>
<head>
<script type="text/javascript" src="https://www.google.com/jsapi"></script>
<script type="text/javascript">
google.load("visualization", "1", {packages:["corechart"]});
google.setOnLoadCallback(drawChart);
function drawChart() {
var data = google.visualization.arrayToDataTable([
['Year', 'Sales', { role: 'annotation' }, 'Expenses', { role: 'annotation' }],
['2004', 1000, 1000, 400, 400],
['2005', 1170, 1170, 460, 460],
['2006', 660, 660, 1120, 1120],
['2007', 1030, 1030, 540, 540]
]);
var options = {
title: 'Company Performance',
vAxis: {title: 'Year', titleTextStyle: {color: 'red'}}
};
var chart = new google.visualization.BarChart(document.getElementById('chart_div'));
chart.draw(data, options);
}
</script>
</head>
<body>
<div id="chart_div" style="width: 900px; height: 500px;"></div>
</body>
</html>
After running this code, you will see the following screen.
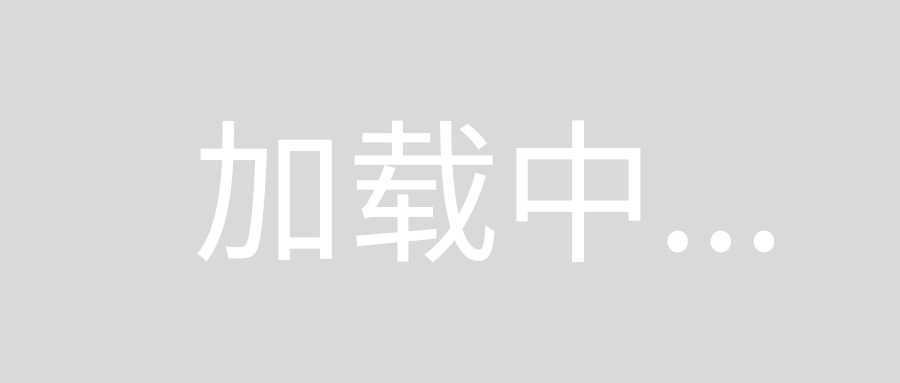
You cannot do this with current functionality as asgallant points out in the comments. If this is an absolute must, then you can parse through the SVG to create line breaks (which have spotty functionality as well). You can see this answer for more information on how to add line breaks in SVG:
How to linebreak an svg text within javascript?
What I've found is that while it isn't possible to have multi-line annotations, you can make html tooltips and annotations: https://developers.google.com/chart/interactive/docs/customizing_tooltip_content. So it is possible to have multi-line annotations.