I have a custom GUI module which uses objects in a tree to manage the interface, and blits them in the right order above one another.
Now amongst my objects, I have some which are just surfaces with per-pixel transparency, and the others use a colorkey.
My problem is that when blitting a surface with per-pixel transparency on another filled with a colorkey, the first surface changes the color of some of the pixels in the second, which means they are no longer transparent. How could I mix those without having to get rid of the per-pixel transparency ?
You could just convert your Surfaces
that use a colorkey to use per-pixel transparency before blitting another per-pixel transparency Surface
on it using convert_alpha
.
Example:
COLORKEY=(127, 127, 0)
TRANSPARENCY=(0, 0, 0, 0)
import pygame
pygame.init()
screen = pygame.display.set_mode((200, 200))
for x in xrange(0, 200, 20):
pygame.draw.line(screen, (255, 255, 255), (x, 0),(x, 480))
# create red circle using a colorkey
red_circle = pygame.Surface((200, 200))
red_circle.fill(COLORKEY)
red_circle.set_colorkey(COLORKEY)
pygame.draw.circle(red_circle, (255, 0, 0), (100, 100), 25)
#create a green circle using alpha channel (per-pixel transparency)
green_circle = pygame.Surface((100, 100)).convert_alpha()
green_circle.fill(TRANSPARENCY)
pygame.draw.circle(green_circle, (0, 255, 0, 127), (50, 50), 25)
# convert colorkey surface to alpha channel surface before blitting
red_circle = red_circle.convert_alpha()
red_circle.blit(green_circle, (75, 75))
screen.blit(red_circle, (0, 0))
pygame.display.flip()
Result:
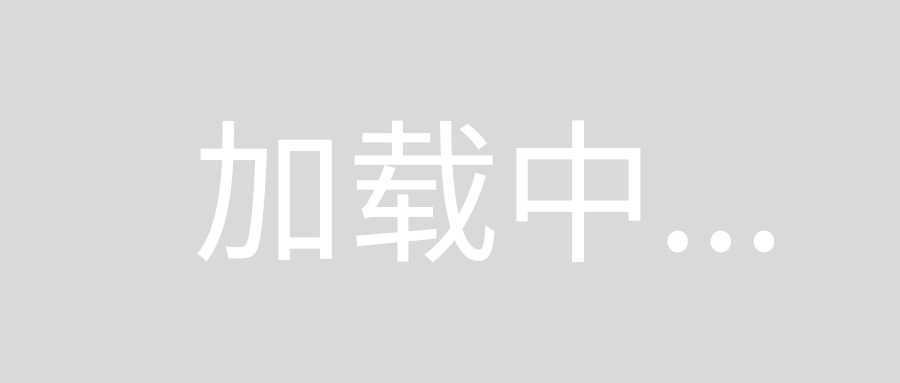