I need to update the sale price programmatically, on variable product and all his variations.
What kind of meta field do I need to add?
I'm trying to update main product such as:
update_post_meta($post_id, '_regular_price', '100');
update_post_meta($post_id, '_price', '50');
update_post_meta($post_id, '_sale_price', '50');
and then I update every single variations
update_post_meta($variation_id, '_regular_price', '100');
update_post_meta($variation_id, '_price', '50');
update_post_meta($variation_id, '_sale_price', '50');
update_post_meta($variation_id, 'attribute_pa_taglia', $term_slug);
update_post_meta($variation_id, '_stock', $stock);
update_post_meta($variation_id, '_stock_status', 'instock');
update_post_meta($variation_id, '_manage_stock', 'yes');
Back end: product detail, everything ok
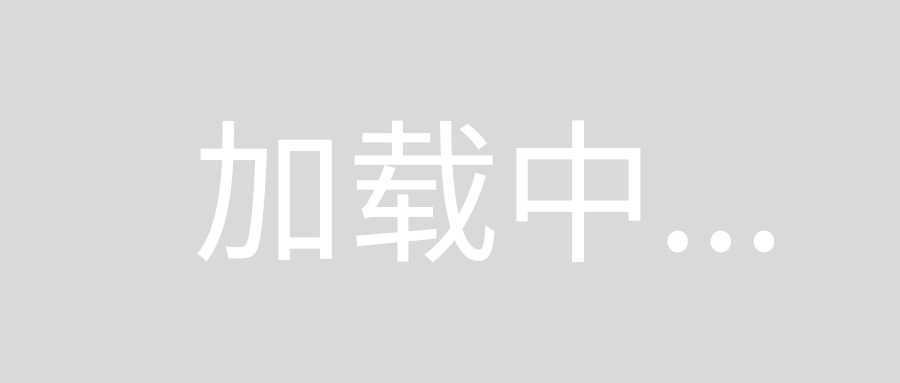
However backend (product list) and frontend get me old price
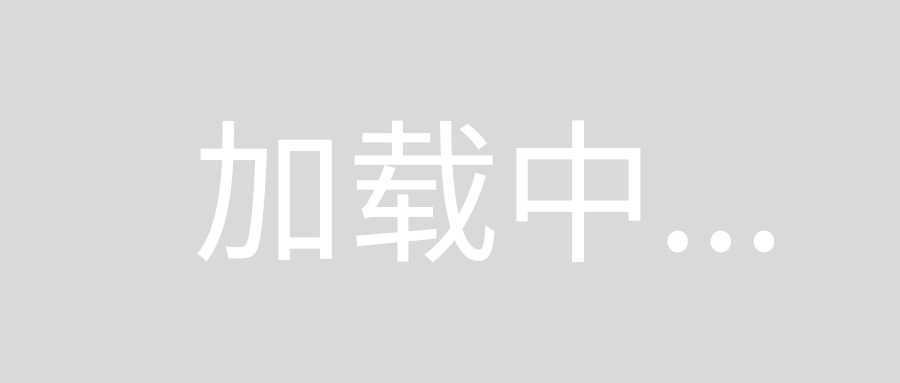
Update: Prices are also cached in transient wp_options
table.
Let say your product ID is 222
, you will have that transients meta_keys in wp_options
table (for this product ID):
'_transient_timeout_wc_product_children_22'
'_transient_wc_product_children_22'
'_transient_timeout_wc_var_prices_222' // <=== <=== HERE
'_transient_wc_var_prices_222' // <=== <=== <=== HERE
What you can try to do is to update expiration date meta_value
to an outdated timestamp, this way:
// Set here your product ID
$main_product_id = 222
$transcient_product_meta_key = '_transient_wc_var_prices_'. $main_product_id;
update_option( $transcient_product_meta_key, strtotime("-12 hours") );
wp_cache_delete ( 'alloptions', 'options' ); // Refresh caches
This way you will force the system to rebuild this outdated cached transient.
Additionally you should try to add/update in your parent product ID (the main product where variation are set) these:
// Set here your Main product ID (for example the last variation ID of your product)
$post_id = 22;
// Set here your variation ID (for example the last variation ID of your product)
$variation_id = 24;
// Here your Regular price
$reg_price = 100;
// Here your Sale price
$sale_price = 50;
update_post_meta($post_id, '_min_variation_price', $sale_price);
update_post_meta($post_id, '_max_variation_price', $sale_price);
update_post_meta($post_id, '_min_variation_regular_price', $reg_price);
update_post_meta($post_id, '_max_variation_regular_price', $reg_price);
update_post_meta($post_id, '_min_variation_sale_price', $sale_price);
update_post_meta($post_id, '_max_variation_sale_price', $sale_price);
update_post_meta($post_id, '_min_price_variation_id', $variation_id);
update_post_meta($post_id, '_max_price_variation_id', $variation_id);
update_post_meta($post_id, '_min_regular_price_variation_id', $variation_id);
update_post_meta($post_id, '_max_regular_price_variation_id', $variation_id);
update_post_meta($post_id, '_min_sale_price_variation_id', $variation_id);
update_post_meta($post_id, '_max_sale_price_variation_id', $variation_id);
// Optionally
wc_delete_product_transients($variation_id);
Furthermore, I've find other solution that work same:
$product_variable = new WC_Product_Variable($post_id);
$product_variable->sync($post_id);
wc_delete_product_transients($post_id);