In my UITableView
that I have setup using Storyboards, I need to be able to add a tool bar that sticks to the bottom of the view, it should not scroll.
Unlike this question: LINK I don't think I could add a TableView subview to a normal view and then just add a toolbar programmatically because I am using dynamic cells which seem a lot easier to integrate via Storyboards.
For now, this is what I am stuck with....
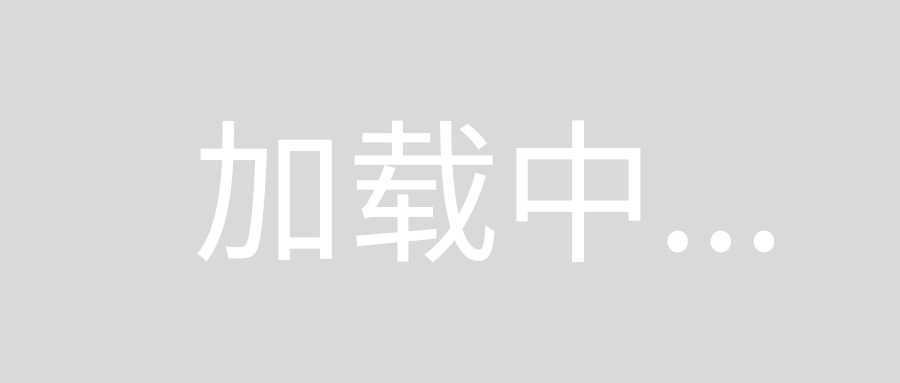
if you want show toolbar in one view controller which placed in some navigation controller.
- select view controller in storyboard
- in utilities, show "attribute inspector". select "bottom bar" style.
- add bar button item
- add code in view controller, to show and hide toolbar:
code:
- (void)viewWillAppear:(BOOL)animated
{
[self.navigationController setToolbarHidden:NO animated:YES];
}
- (void)viewWillDisappear:(BOOL)animated
{
[self.navigationController setToolbarHidden:YES animated:YES];
}
Very easy. Just click on the navigation controller. Then in Show Attributes Inspector then navigation controller then click on the shows toolbar.
Check the screen shot.
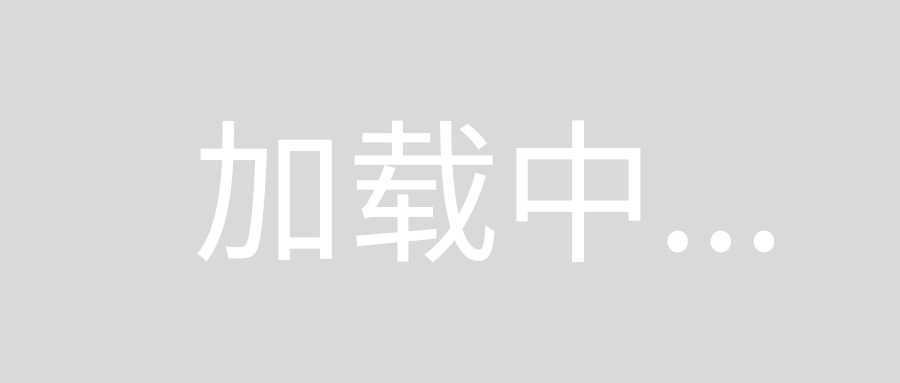
For Swift users, you can use the following code:
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated);
self.navigationController?.setToolbarHidden(false, animated: animated)
}
override func viewWillDisappear(animated: Bool) {
super.viewWillDisappear(animated);
self.navigationController?.setToolbarHidden(true, animated: animated)
}
This remedy works for (2016) iOS 9.2.
We all hate how Apple makes us waste time in stuff that should be straightforward like this. I like step by step solutions for this type of silly problems, so I will share it with you!:
- Select your View controller > Attribute Inspector > Select "Opaque
Toolbar"
- Now, drag and drop a "Bar Button item to your Storyboard.
- Select your newly dropped Bar Button Item > Atrribute Inspector >
System Icon > Select your favorite icon.
In the viewDidLoad() method of your View controller, add this code before anything else:
override func viewDidLoad(animated: Bool) {
self.navigationController?.setToolbarHidden(false, animated: true)
//the rest of code
}
You don't want that toolbar hanging around elsewhere, so add this inside your view to
hide it once the current window is dismissed:
-
override func viewWillDisappear(animated: Bool) {
super.viewWillDisappear(animated);
self.navigationController?.setToolbarHidden(true, animated: animated)
}
Voila!
- Drag a UIViewController into Storyboard
- Drag a UIToolbar on top of the Storyboard's contents.
- Drag a UITableView on top of the Storyboard's contents.
- Link the tableview's delegate and datasource to your source code.
Although you won't be able to use UITableViewController as your linking class step 4 will allow you to link it to a regular UIViewController.
You'll need something like this in the header though
@interface MyViewController : UIViewController <UITableViewDelegate, UITableViewDataSource>
It'll look something like this in your storyboard:
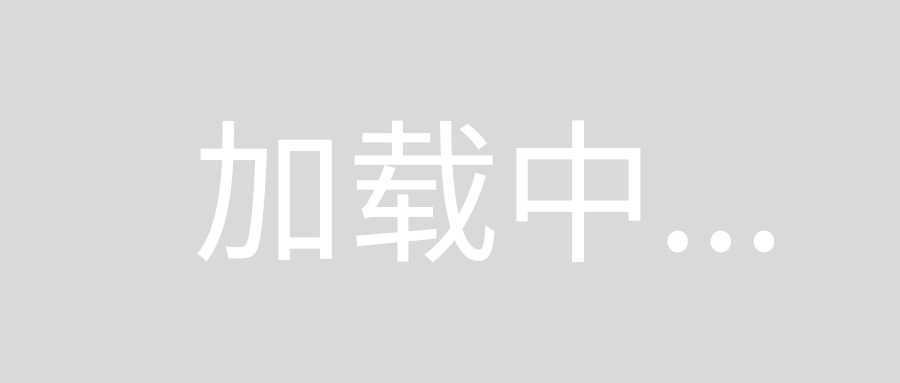
I used an intermediate View Controller with a Container view to the table. Add the toolbar view to the intermediate, and make it look however you want (use UIButtons instead of UIBarButtonItem).
If you do this, have the container view stretch to the top of the screen and not the bottom of the nav bar or you'll pull your hair out trying to get the scroll insets right.
Some more details in a similar question https://stackoverflow.com/a/31878998/1042111