I am using JFreeChart 1.0.9 to create a chart, and iText 1.3.1 to display this chart in a PDF file.
To do so, I am creating a JFreeChart, and then converting it to a byte array :
final TimeSeriesCollection dataset = new TimeSeriesCollection();
final TimeSeries s1 = new TimeSeries("Series 1", Minute.class);
s1.add(new Minute(0, 0, 7, 12, 2003), 1.2);
s1.add(new Minute(30, 12, 7, 12, 2003), 3.0); // 12h30 => value = 3
s1.add(new Minute(15, 14, 7, 12, 2003), 8.0);
final TimeSeries s2 = new TimeSeries("Series 2", Minute.class);
s2.add(new Minute(0, 3, 7, 12, 2003), 0.0);
s2.add(new Minute(30, 9, 7, 12, 2003), 0.0);
s2.add(new Minute(15, 10, 7, 12, 2003), 0.0);
dataset.addSeries(s1);
dataset.addSeries(s2);
XYDataset dataset2 = dataset;
final JFreeChart chart = ChartFactory.createTimeSeriesChart(
"Sample Chart",
"Date",
"Value",
dataset2,
true,
true,
false
);
chart.setBackgroundPaint(Color.white);
ChartRenderingInfo info = new ChartRenderingInfo(new StandardEntityCollection());
BufferedImage originalImage = chart.createBufferedImage(500, 300, info);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ImageIO.write( originalImage, "jpg", baos );
byte[] imageInByte = baos.toByteArray();
Then I am simply putting this byte array in the PDF file using iText as follow :
Document document=new Document();
PdfWriter.getInstance(document,new FileOutputStream("hello.pdf"));
document.open();
Image image = Image.getInstance(imageInByte);
document.add(image);
document.close();
But when I open my PDF file, the area where my image should be is all black.
Do you have an idea of what could be the cause of it?
It looks like the image is being inverted during the conversion process. Try the following code
package demo;
import java.awt.Color;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.JFreeChart;
import org.jfree.data.time.Minute;
import org.jfree.data.time.TimeSeries;
import org.jfree.data.time.TimeSeriesCollection;
import org.jfree.data.xy.XYDataset;
import com.lowagie.text.Document;
import com.lowagie.text.DocumentException;
import com.lowagie.text.pdf.PdfWriter;
public class PDF {
public static void main(String[] args) {
final TimeSeriesCollection dataset = new TimeSeriesCollection();
final TimeSeries s1 = new TimeSeries("Series 1", Minute.class);
s1.add(new Minute(0, 0, 7, 12, 2003), 1.2);
s1.add(new Minute(30, 12, 7, 12, 2003), 3.0); // 12h30 => value = 3
s1.add(new Minute(15, 14, 7, 12, 2003), 8.0);
final TimeSeries s2 = new TimeSeries("Series 2", Minute.class);
s2.add(new Minute(0, 3, 7, 12, 2003), 0.0);
s2.add(new Minute(30, 9, 7, 12, 2003), 0.0);
s2.add(new Minute(15, 10, 7, 12, 2003), 0.0);
dataset.addSeries(s1);
dataset.addSeries(s2);
XYDataset dataset2 = dataset;
final JFreeChart chart = ChartFactory.createTimeSeriesChart(
"Sample Chart",
"Date",
"Value",
dataset2,
true,
true,
false
);
chart.setBackgroundPaint(Color.white);
java.awt.Image originalImage = chart.createBufferedImage(500, 300);
try {
Document document=new Document();
PdfWriter.getInstance(document,new FileOutputStream("hello.pdf"));
document.open();
com.lowagie.text.Image image1 = com.lowagie.text.Image.getInstance(originalImage,Color.white);
document.add(image1);
document.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (DocumentException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Using the latest version of iText You I get this PDF
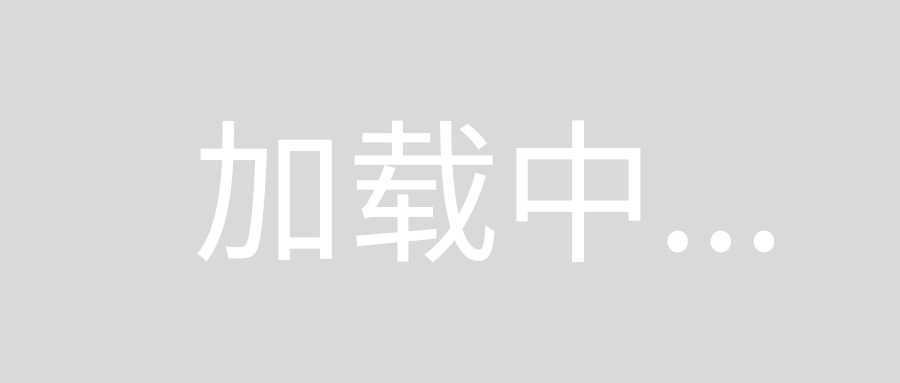
hopefully you will get the same
I have made the follwing changes to you code
java.awt.Image originalImage = chart.createBufferedImage(500, 300);
having Removed
ChartRenderingInfo info = new ChartRenderingInfo(new StandardEntityCollection());
As its not needed. I've also removed the ByteArrayOutputStream
and replaced it with
com.lowagie.text.Image image1 = com.lowagie.text.Image.getInstance(originalImage,Color.white);
Note the use of full names for com.lowagie.text.Imag
e and java.awt.Image
as both AWT and Lowagie have classes called Image.