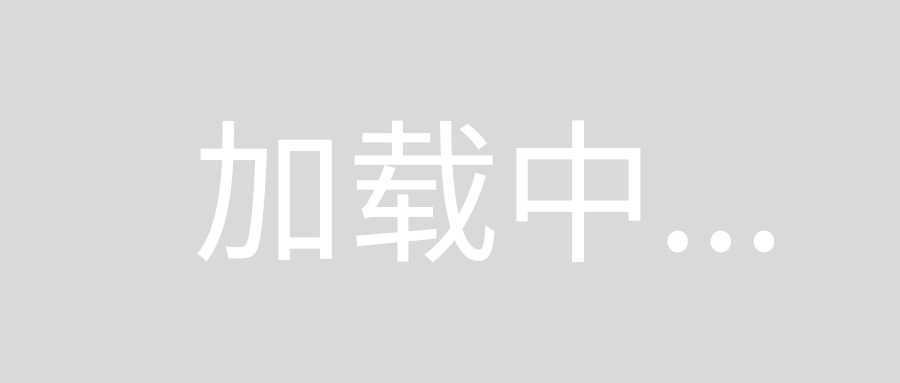
am using navigation bar programmatically in swift, but am not able to show the bar button items in navigation bar,
this is the code what I did
override func viewDidLoad() {
super.viewDidLoad()
let navBar: UINavigationBar = UINavigationBar(frame: CGRect(x: 0, y: 0, width: 420, height: 65))
self.view.addSubview(navBar)
navBar.backgroundColor = hexStringToUIColor("4DC8BD")
let navigationItem = UINavigationItem()
self.title = "Transport APP"
let btn1 = UIButton(type: .custom)
btn1.setImage(UIImage(named: "Menu1"), for: .normal)
btn1.frame = CGRect(x: 30, y: 30, width: 30, height: 30)
btn1.addTarget(self, action: #selector(HomeViewController.menubuttonclick(_:)), for: .touchUpInside)
let item1 = UIBarButtonItem(customView: btn1)
self.navigationItem.setRightBarButtonItems([item1], animated: true)
}
@IBAction func menubuttonclick(_ sender:UIBarButtonItem )
{
print("this menu button click")
}
I can try many ways but am not getting the results
how to show show bar button item in navigation bar,
You should add UINavigationItem
to your UINavigationBar
and in item1 need to be added in navitem Look at below code
let navitem = UINavigationItem()
navitem.rightBarButtonItem = item1
navBar.setItems([navitem], animated: true)
While the other mentioned solutions definitely work for programmatically defining the navigation item, some would prefer a storyboard solution. I searched for a Swift 4, Xcode 9 storyboard solution and was unable to find one, so I will show my solution.
Here is a screenshot of my storyboard before adding the bar button item.
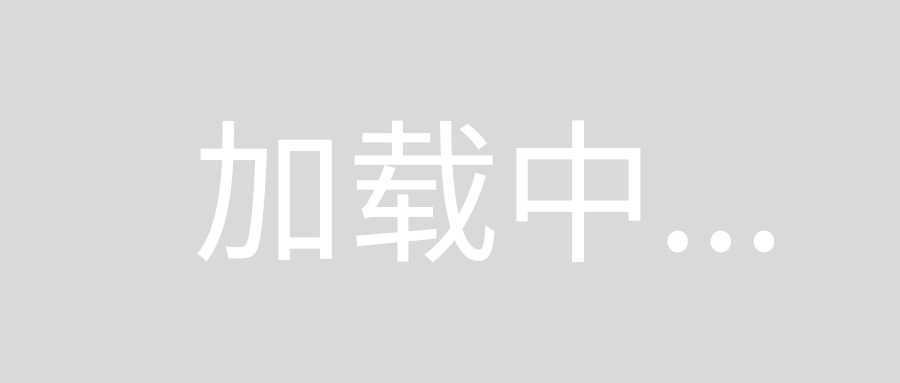
The issue I was having is that while the Shops tableview is embedded in the navigation controller, and adding a bar button item was no issue; the Employees tableview is pushed via the navigation controller in the didSelectRowAt function.
extension ShopsViewController: UITableViewDelegate {
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
let selectedShop = fetchedResultsController.object(at: indexPath)
let st = UIStoryboardname: "Main", bundle: Bundle.main)
let vc = st.instantiateViewController(withIdentifier: "EmployeeViewController") as! EmployeeViewController
vc.shop = selectedShop
self.navigationController?.pushViewController(vc, animated: true)
}
}
The result is that I could not drag a bar button item from the storyboard. When I tried, the item would end up in the tab bar at the bottom.
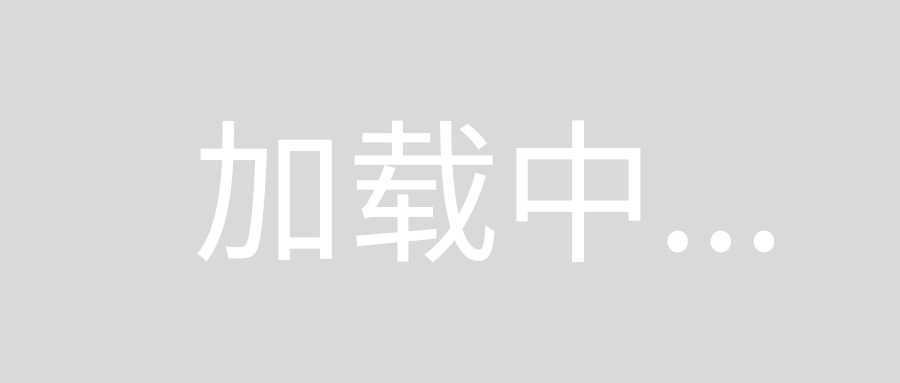
I found an article that suggested embedding the second view controller in a navigation controller, but that adds other levels of complexity that I wanted to avoid. The work around I found is to drag a navigation item to the navigation bar area, and then you can add a bar button item with no problems.
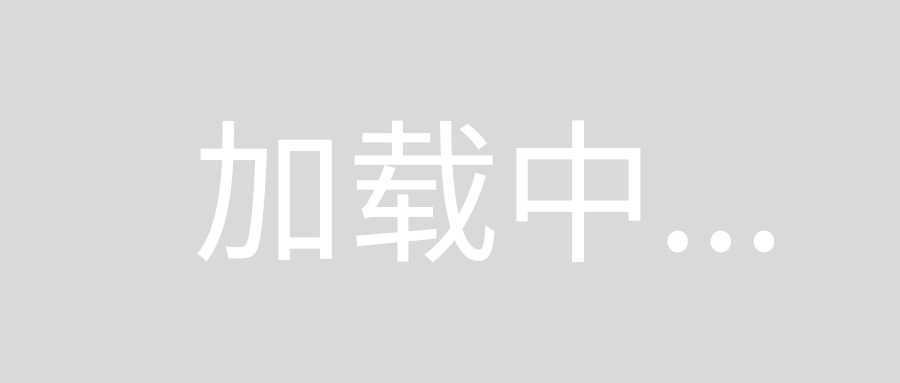
Here is the result: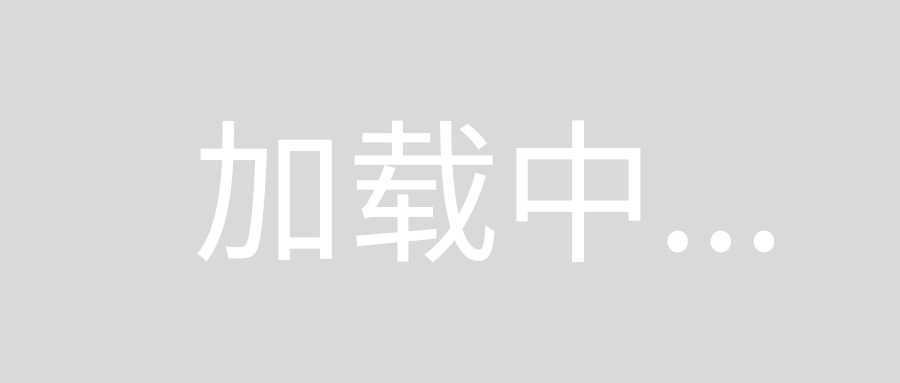
I know there is a lot of debate whether storyboards or programmatic layout is better. While I am still very much a beginning iOS developer and cannot personally speak to that, I am finding that sometimes the storyboard solution fits the problem best. I hope this helps other beginners.
Swift 3+: Define the barbutton.
//:: Left bar items
lazy var leftBarItem: Array = { () -> [UIBarButtonItem] in
let btnBack = UIButton(type: .custom)
btnBack.frame = kBAR_FRAME
btnBack.addTarget(self, action: #selector(clickOnBackBtn(_:)), for: .touchUpInside)
let item = UIBarButtonItem(customView: btnBack)
item.tag = 3
return [item]
}()
Add this line into viewDidLoad
self.navigationItem.setLeftBarButtonItems(self.leftBarItem, animated: true)
Bar Button Action
@objc func clickOnBackBtn(_ sender: Any){
}
if you've already created UINavigationController()
you can set navigation items using self.navigationItem
like this (in viewDidLoad
function):
self.navigationItem.title = "Transport APP"
self.navigationItem.rightBarButtonItem = item1
but if you need to know how to create UINavigationController()
for a view, you can do this in AppDelegate
class:
let myView = ... //initial your view controller
let nav = UINavigationController()
nav.viewControlles = [myView]
and then everywhere you need, you should push nav view.