i want to create a new layer using scapy,i created a new layer but when i sent it to another computer it got lost, wireshark also cant recognize it.
how can i slove this problem?
class OMER(Packet):
name = "OMER"
fields_desc = [StrLenField("Omer", "", None)]
When you create a new protocol or a new layer with scapy
, other network tools like wireshark
(and others) since they are not aware of your protocol's specifics will not be able to automatically parse it correctly.
If you want to experiment with a new protocol you will have to create your own local decoder. The following example even its minimal, it demonstrates all of the above:
#!/usr/bin/env python
from scapy.all import *
# create a simple protocol
# (example similar with the one in the scapy docs...)
class Exmpl(Packet):
name = "myprotocol"
fields_desc=[ ShortField("fld1",5),
XByteField("fld2",3) ]
from scapy.utils import PcapWriter
# write data in a pcap file to examine later with
# 1 -> scapy
# 2 -> wireshark
print '\n[+] Writing net.pcap file...'
cap = PcapWriter("net.pcap", append=True, sync=True)
for i in range(10):
packet = Exmpl(fld1=i)
cap.write(packet)
# read the data and examine them with scapy
# scapy is aware of the "Exmpl" protocol (e.g. its fields etc...)
# and how to decode it, while wireshark is not
print '[+] Examining net.pcap file...\n'
packets = rdpcap('net.pcap')
for p in packets:
Exmpl(str(p)).show()
The output of the above script will be like:
[+] Writing net.pcap file...
[+] Examining net.pcap file...
###[ myprotocol ]###
fld1 = 0
fld2 = 0x3
###[ myprotocol ]###
fld1 = 1
fld2 = 0x3
###[ myprotocol ]###
fld1 = 2
fld2 = 0x3
...skip...
As you can see scapy is aware of the protocol and thus can parse the data correctly. Now if you try to examine the "net.pcap" file with wireshark
you will see the following:
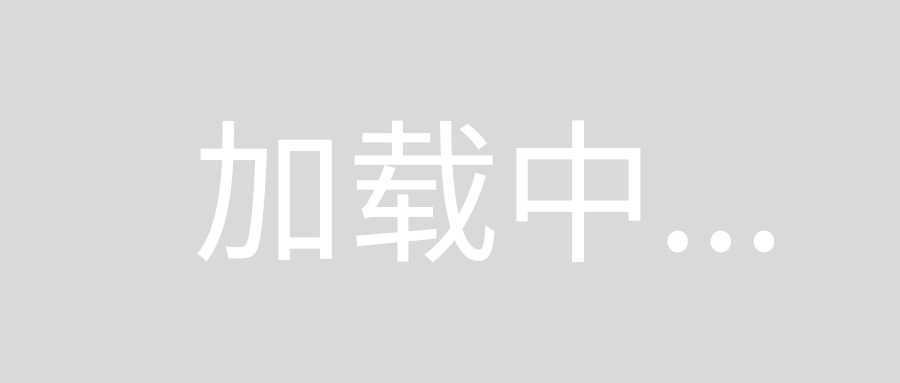
wireshark is not aware of your protocol and as a result it can't parse it correctly.
Notice: As you can understand, even if you send those packets in another device (to actually do that you'll have to implement some other stuff also) then that device must also be aware of your protocol, otherwise it won't be able to parse it correctly. That is why when you tried to send the packets from one computer to another, the receiver couldn't successfully decode them.
Did you bind the new layer/protocol to the previous layer/protocol? E.g. bind_layers(Ether, OMER) if the OMER layer comes right after the Ether layer.
You have made your custom layer. However you shoukd keep in your mind that this custom layer wouldnt be recognized by a operating system untill you write networking at kernel level and that must be present in every operating system. So on the other host, you write another python script that tells operating system to disect the packet containing your custom layer accordingly. Write your own sniffer packet and then initliaze your custom layer class so that while sniffing you actually make use of that class while disecting the packet and then you can view the packet with your custom layer.
Note that you need to bind the layer, your new layer with the layer in froth of it. It appears as if you have constructed right packet, however when it reaches the other host it simply cannot decode untill you bind the layer. Only when you bind it, your operating system knows to disect the packet excatly after your ip layer or udp layer etc... Have been dissected .
Also in oder to squeeze your custom layer between two well known protocols, say for example udp with port 53, operating by default binds dns to udp port 53. If you ever wanted to inject your new layer between udp and dns say, u need to firstly unbind udp and dns and then say to udp that I want my custom layer to be followed instead of dns layer. Just use split_layers to unbind udp and dns and then bind your custom layer to udp and also bind your dns to your new custom layer.
Thinks of it as learning a foreign language. Let's say your family is speaking only english.
- You learn chinese and now are able to say sentences in chinese (that's what you did by creating the layer in scapy and building packets)
- Your family can hear the chinese sentences (wireshark can receive the packets)
- But your family cannot understand the sentences since they will still just speak english (wireshark cannot dissects the packets as it does not know your protocol)
- If you abolutely want your family to understand you when speaking chinese, then you have to teach them (implement the dissector in wireshark)
Hope that's clear :-)