I have a texture brush that uses a certain image to make the texture to be displayed like this:
Image image = new Bitmap("Untitled.png");
for (int i = 0; i < points.Count; i++)
{
using (TextureBrush tbr = new TextureBrush(image))
{
tbr.RotateTransform(i * 4);
var p = PointToClient(Cursor.Position);
tbr.Transform = new Matrix(
75.0f / 640.0f,
0.0f,
0.0f,
75.0f / 480.0f,
0.0f,
0.0f);
e.Graphics.FillEllipse(tbr, p.X - 50, p.Y - 50, 100, 100);
Pen p3 = new Pen(tbr);
e.Graphics.DrawEllipse(Pens.DeepSkyBlue, p.X - 50, p.Y - 50, 100, 100);
}
}
and here's the image it is using:
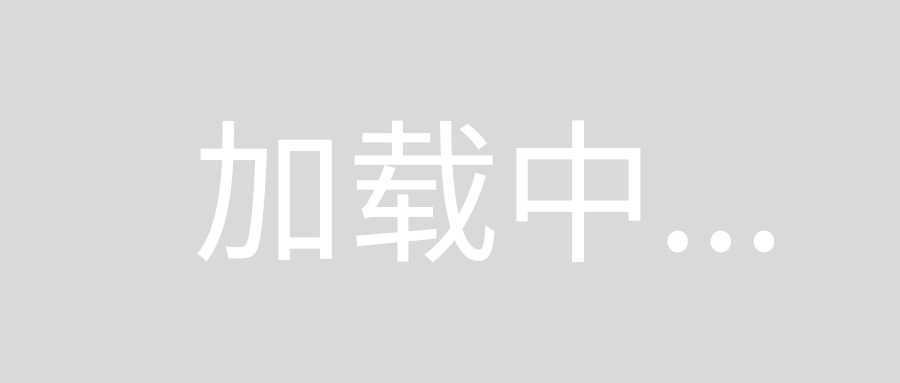
This is how it turns out:
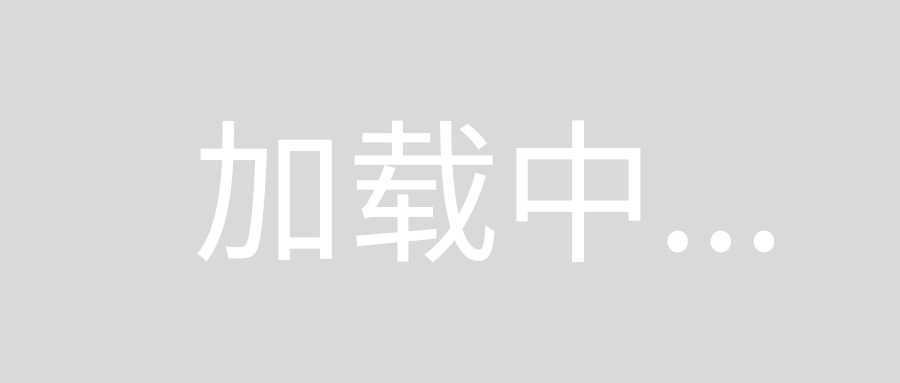
I want the image to fill the circle so that it looks like this(edited image):
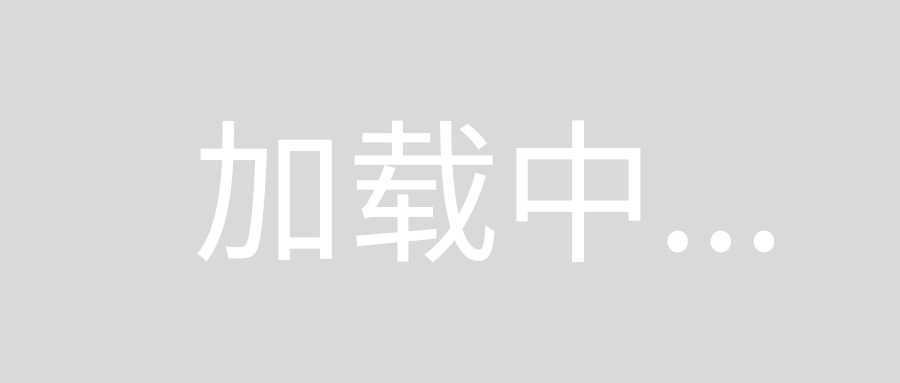
Any Help would be appreciated.
You need to scale using the correct numbers.
If you want an image with a size = width * height pixels to fill a circle of diameter you should scale like this:
int diameter = 100;
Image image = new Bitmap(yourImage);
float scaleX = 1f * diameter / image.Size.Width;
float scaleY = 1f * diameter / image.Size.Height;
Note however that your TextureBrush
will always reveal a tiling made from your image. This seemed ok for your original question, especially when rotating the images in the tail to get rid of any artifacts.
But here it may simply not be what you want.
If you want the image to follow the mouse you need to draw it.
Here is an example, that uses a checkbox to switch between tiling and drawing. The animation uses only one frame:
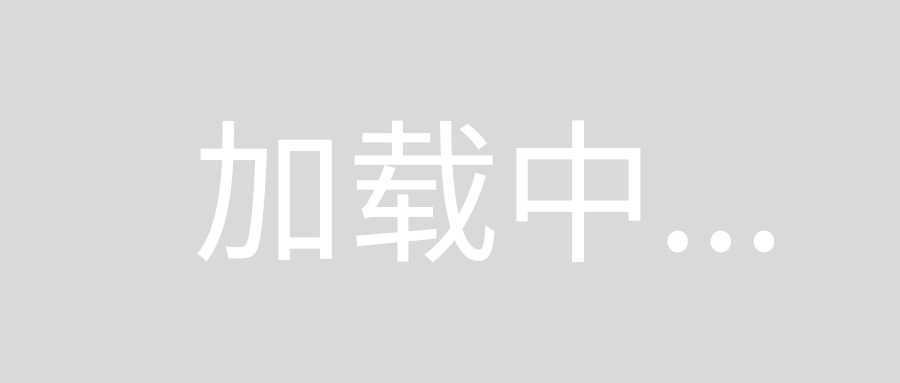
for (int i = 0; i < points.Count; i++)
{
using (TextureBrush tbr = new TextureBrush(image))
{
tbr.RotateTransform(i * 4); // optional
var p = PointToClient(Cursor.Position);
tbr.Transform = new Matrix(
scaleX,
0.0f,
0.0f,
scaleY,
0.0f,
0.0f);
// any tile mode will work, though not all the same way
tbr.WrapMode = WrapMode.TileFlipXY;
if (cbx_tileFunny.Checked)
e.Graphics.FillEllipse(tbr, p.X - diameter/2,
p.Y - diameter/2, diameter, diameter);
else
{
((Bitmap)image).SetResolution(e.Graphics.DpiX, e.Graphics.DpiY); // (**)
e.Graphics.ScaleTransform(scaleX, scaleY);
e.Graphics.DrawImage( image, (p.X - diameter/2) / scaleX,
(p.Y - diameter/2 ) / scaleY);
e.Graphics.ResetTransform();
}
/// ? Pen p3 = new Pen(tbr);
e.Graphics.DrawEllipse(Pens.DeepSkyBlue, p.X - diameter/2,
p.Y - diameter/2, diameter, diameter);
}
}
Do note the extra scaling needed here (**) if the image has a different dpi setting than your screen.
Also: While it is usually a good idea to create and dispose Pens and Brushes quickly, when so much effort is put into creating the Brush and/or Image caching them or even a series of them seems rather preferrable, imo.