function [J, grad] = costFunction(theta, X, y)
m = length(y);
h = sigmoid(X*theta);
sh = sigmoid(h);
grad = (1/m)*X'*(sh - y);
J = (1/m)*sum(-y.*log(sh) - (1 - y).*log(1 - sh));
end
I'm trying to compute the cost function for logistic regression. Can someone please tell me why this isn't accurate?
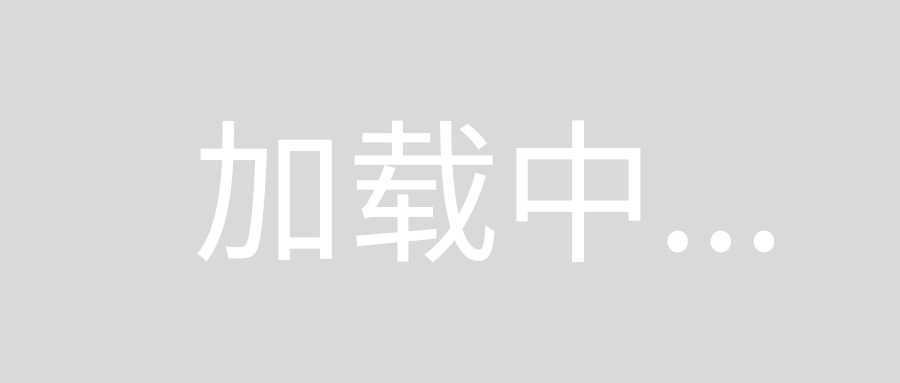
Update: Sigmoid function
function g = sigmoid(z)
g = zeros(size(z));
g = 1./(1 + exp(1).^(-z));
end
As Dan stated, your costFunction calls sigmoid twice. First, it performs the sigmoid function on X*theta
; then it performs the sigmoid function again on the result of sigmoid(X*theta)
. Thus, sh = sigmoid(sigmoid(X*theta))
. Your cost function should only call the sigmoid function once.
See the code below, I removed the sh
variable and replaced it with h
everywhere else. This causes the sigmoid function to only be called once.
function [J, grad] = costFunction(theta, X, y)
m = length(y);
h = sigmoid(X*theta);
grad = (1/m)*X'*(h - y);
J = (1/m)*sum(-y.*log(h) - (1 - y).*log(1 - h));
end