I want to create spring boot web application.
I have two static html files: one.html, two.html.
I want to map them as follows
localhost:8080/one
localhost:8080/two
without using template engines (Thymeleaf).
How to do that? I have tried many ways to do that, but I have 404 error or 500 error (Circular view path [one.html]: would dispatch back to the current handler URL).
OneController.java is:
@Controller
public class OneController {
@RequestMapping("/one")
public String one() {
return "static/one.html";
}
}
Project structure is
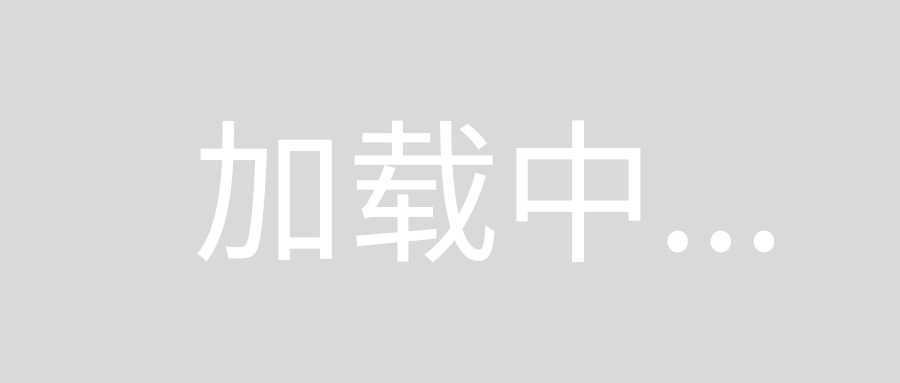
Please update your WebMvcConfig and include UrlBasedViewResolver and /static resource handler. Mine WebConfig class looks as follows:
@Configuration
@EnableWebMvc
public class WebConfig extends WebMvcConfigurerAdapter {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/static/**").addResourceLocations("classpath:/static/");
super.addResourceHandlers(registry);
}
@Bean
public ViewResolver viewResolver() {
UrlBasedViewResolver viewResolver = new UrlBasedViewResolver();
viewResolver.setViewClass(InternalResourceView.class);
return viewResolver;
}
}
I have checked it and seems working.
Maciej's answer is based on browser's redirect. My solution returns static without browser interaction.
In my case I want to map all sub paths to the same file but keep the browser path as the original requested, at same time I use thymeleaf then I don't want to override it's resolver.
@Controller
public class Controller {
@Value("${:classpath:/hawtio-static/index.html}")
private Resource index;
@GetMapping(value = {"/jmx/*", "/jvm/*"}, produces = MediaType.TEXT_HTML_VALUE)
@ResponseBody
public ResponseEntity actions() throws IOException {
return ResponseEntity.ok(new InputStreamResource(index.getInputStream()));
}
}
Obs. every hit will read the data from the index.html file, it will not be cached
If you don't care about additional browser redirect you can use this:
@Controller
public class OneController {
@RequestMapping("/one")
public String one() {
return "redirect:/static/one.html";
}
}