My security team reported one html tag injection security issue for the below code
function ClosePopUp(objBhID) {
var pageName = window.location.pathname;
var modalPopupBehavior = $find(objBhID);
if (modalPopupBehavior != null && modalPopupBehavior != 'undefined') {
modalPopupBehavior.hide();
}
if (objBhID == 'bhThankMsg' && pageName == '/Projects/Comm.aspx') {
var objPartnerID = '<%=Request.QueryString["partnerid"]%>';
if (objPartnerID != 'undefined' && objPartnerID != null && objPartnerID != '') {
window.location = '/Projects/Comm.aspx?Id=<%=Request.QueryString["ID"]%>&partnerid=<%=Request.QueryString["partnerid"]%>';
}
else {
window.location = '/Projects/Comm.aspx?Id=<%=Request.QueryString["ID"]%>';
}
}
}
My security team reported the below issue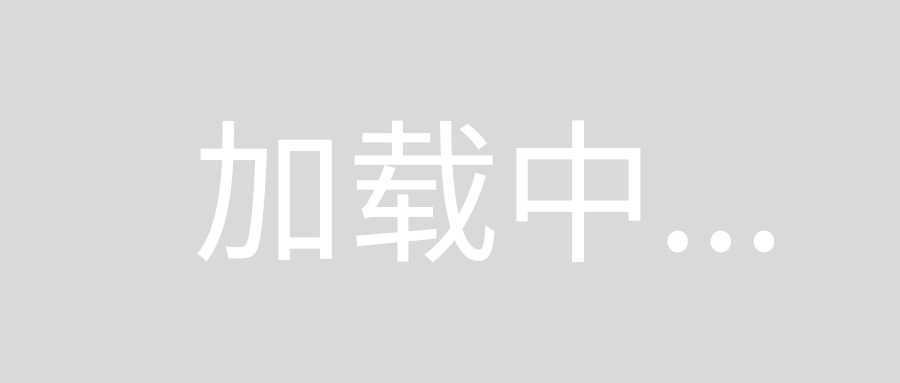
HTML tag injection vulnerabilities were identified on this web application. HTML tag injections are used to aid in Cross-Site Request Forgeries and phishing attacks against third-party web sites, and can often double as Cross-Site Scripting vulnerabilities. Recommendations include implementing secure programming techniques that ensure proper filtration of user-supplied data, and encoding all user supplied data to prevent inserted scripts being sent to end users in a format that can be executed.
My requirement is i don't want to allow any user or hacker to inject unnecessary data, how to achieve this ?
Although the other answer will work in this case for URLs, because the built in URL encoding happens to encode html and Javascript escape characters, this is not a complete solution for using dynamic server-side values within Javascript. Therefore I post my answer here so hopefully no one falls into the trap of using the wrong encoding and produces something insecure.
The OWASP rule is:
Except for alphanumeric characters, escape all characters less than
256 with the \xHH format to prevent switching out of the data value
into the script context or into another attribute. DO NOT use any
escaping shortcuts like \" because the quote character may be matched
by the HTML attribute parser which runs first. These escaping
shortcuts are also susceptible to "escape-the-escape" attacks where
the attacker sends \" and the vulnerable code turns that into \"
which enables the quote.
However, an easier, and imo simpler solution is to never put server-side data within any script tag or script enabled attribute at all. Use HTML5 data attributes, then you can insert your data HTML encoded into a safer HTML context.
I see you appear to be using JQuery. Why not do the following, then you can use the built-in ASP.NET encoding functions?
<div id="myDynamicData" data-partnerId="<%=Server.HTMLEncode(Request.QueryString["partnerid"])%>" data-id="<%=Server.HTMLEncode(Request.QueryString["id"]%>)" />
Or simply
<div id="myDynamicData" data-partnerId="<%:Request.QueryString["partnerid"]%>" data-id="<%:Request.QueryString["id"]%>" />
on newer versions of .NET.
Your code then becomes:
function ClosePopUp(objBhID) {
var pageName = window.location.pathname;
var modalPopupBehavior = $find(objBhID);
if (modalPopupBehavior != null && modalPopupBehavior != 'undefined') {
modalPopupBehavior.hide();
}
if (objBhID == 'bhThankMsg' && pageName == '/Projects/Comm.aspx') {
var objPartnerID = $('#myDynamicData').data('partnerId');
var id = $('#myDynamicData').data('id');
if (objPartnerID) {
window.location = '/Projects/Comm.aspx?Id=' + encodeURIComponent(id) + '&partnerid=' + encodeURIComponent(objPartnerID);
}
else {
window.location = '/Projects/Comm.aspx?Id=' + encodeURIComponent(id);
}
}
}
<%= HttpUtility.UrlEncode( Request.QueryString["ID"] ) %>