I know that CloudBlockBlob.DeleteIfExists() returns true if the blob exists and false when it does not.
However I'm curious the know what happens if the blob does exist, but something goes wrong in Azure which causes the file deletion to not occur (I can't find any documentation on that behavior).
My concern is that it will return false instead of throwing some kind of exception, so I'll believe the blob is deleted, when it's actually still there.
In short, if I get a value of false back, does it always mean that the blob didn't exist, no deletion was necessary, and I'll get some kind of exception if something goes wrong on Azure's end?
Thanks.
Looking at the source code for this method here
, you will get true
if the blob is deleted, false
if the blob (or blob container) doesn't exist. In all other circumstances (say the blob is leased and thus can't be deleted), an exception will be raised. Here's the relevant code:
public virtual bool DeleteIfExists(DeleteSnapshotsOption deleteSnapshotsOption = DeleteSnapshotsOption.None, AccessCondition accessCondition = null, BlobRequestOptions options = null, OperationContext operationContext = null)
{
BlobRequestOptions modifiedOptions = BlobRequestOptions.ApplyDefaults(options, BlobType.Unspecified, this.ServiceClient);
operationContext = operationContext ?? new OperationContext();
try
{
this.Delete(deleteSnapshotsOption, accessCondition, modifiedOptions, operationContext);
return true;
}
catch (StorageException e)
{
if (e.RequestInformation.HttpStatusCode == (int)HttpStatusCode.NotFound)
{
if ((e.RequestInformation.ExtendedErrorInformation == null) ||
(e.RequestInformation.ExtendedErrorInformation.ErrorCode == BlobErrorCodeStrings.BlobNotFound) ||
(e.RequestInformation.ExtendedErrorInformation.ErrorCode == BlobErrorCodeStrings.ContainerNotFound))
{
return false;
}
else
{
throw;
}
}
else
{
throw;
}
}
}
I know that CloudBlockBlob.DeleteIfExists() returns true if the blob exists and false when it does not.
If the blob is existing and could be deleted then it returns true.
If the blob is not existing then it will return false.
In short, if I get a value of false back, does it always mean that the blob didn't exist
No, If the blob exists and we can’t delete it then also return false with exception . I test it on my side. If the blob is leased, and we try to delete it then we will get false and 412 exception.
More detail info you could refer to the screenshot.
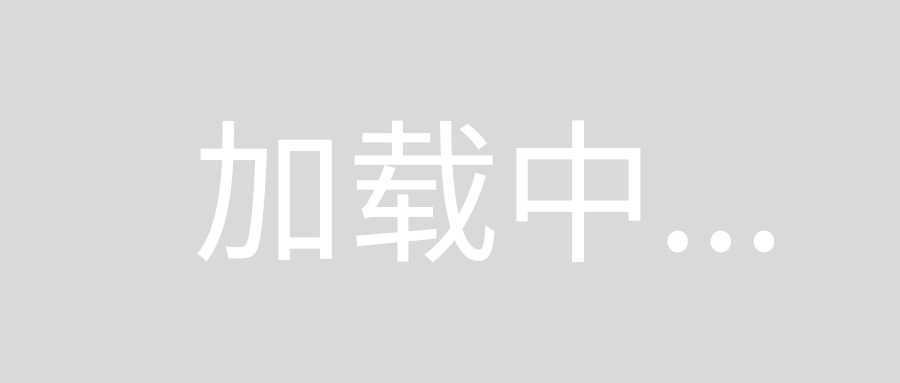