可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
Consider this simple example
library(tidyverse)
tibble(x = as.factor(c('good', 'neutral', 'bad')),
y = as.factor(c('bad', 'neutral', 'bad'))) %>%
ggplot(aes(x = x, y = y)) + geom_point()
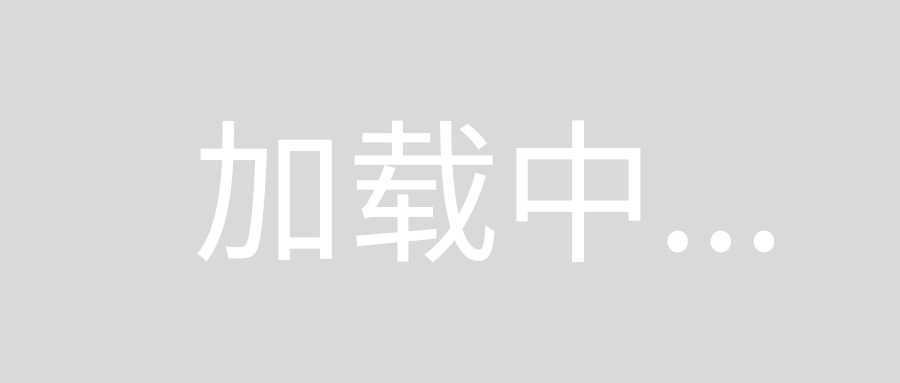
I would like to put the x labels (good
, neutral
, bad
) in different colored boxes. For instance, good
(on both the x and y axis) would be surrounded on a small green box, and so on.
Can I do that in ggplot2
?
回答1:
Solution using geom_label
outside the plot area:
ggplot(data, aes(x, y)) +
geom_point() +
geom_label(aes(0.3, y, label = y, fill = y), hjust = 0) +
geom_label(aes(x, 0.45, label = x, fill = x)) +
theme_minimal() +
theme(
axis.text = element_blank(),
axis.ticks = element_blank(),
legend.position = "none"
) +
coord_cartesian(xlim = c(1, 3), ylim = c(1, 2), clip = "off")
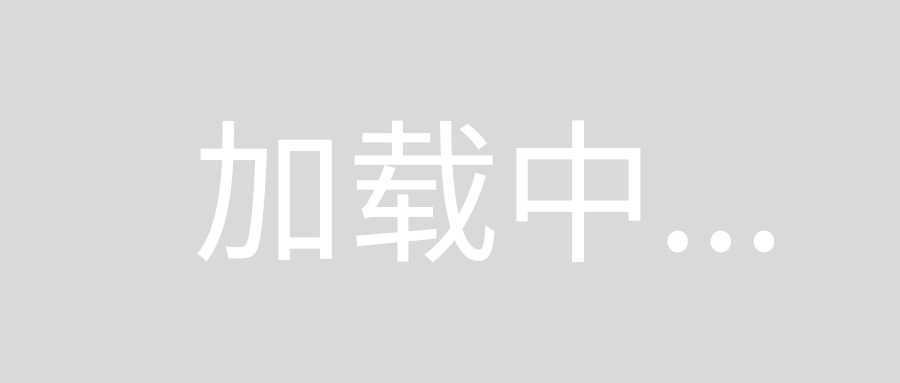
Another solution
You should create geom_rect
with borders, but without fill and plot them outside the plot area (using coord_cartesian
):
library(tidyverse)
data <- tibble(
x = as.factor(c('good', 'neutral', 'bad')),
y = as.factor(c('bad', 'neutral', 'bad'))
)
ggplot(data, aes(x, y)) +
geom_point() +
# put rects on y-axis
geom_rect(aes(xmin = 0.1, xmax = 0.45, color = y,
ymin = as.numeric(y) - 0.1, ymax = as.numeric(y) + 0.1),
fill = NA, size = 3) +
# put rects on x-axis
geom_rect(aes(ymin = 0.3, ymax = 0.4, color = x,
xmin = as.numeric(x) - 0.15, xmax = as.numeric(x) + 0.15),
fill = NA, size = 3) +
# Here it's important to specify that your axis goes from 1 to max number of levels
coord_cartesian(xlim = c(1, 3), ylim = c(1, 2), clip = "off")
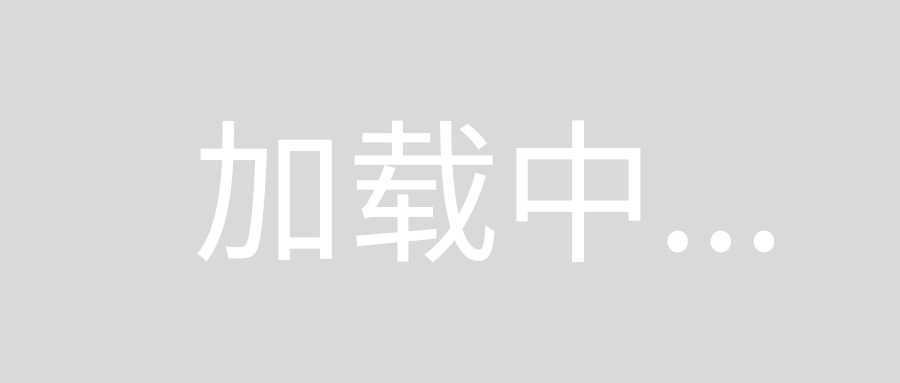
回答2:
Like this?
tibble(x = as.factor(c('good', 'neutral', 'bad')),
y = as.factor(c('bad', 'neutral', 'bad'))) %>%
ggplot(aes(x = x, y = y)) +
geom_point() +
theme(axis.text.x = element_text(color = c('red', 'blue', 'green')))
Your Plot:
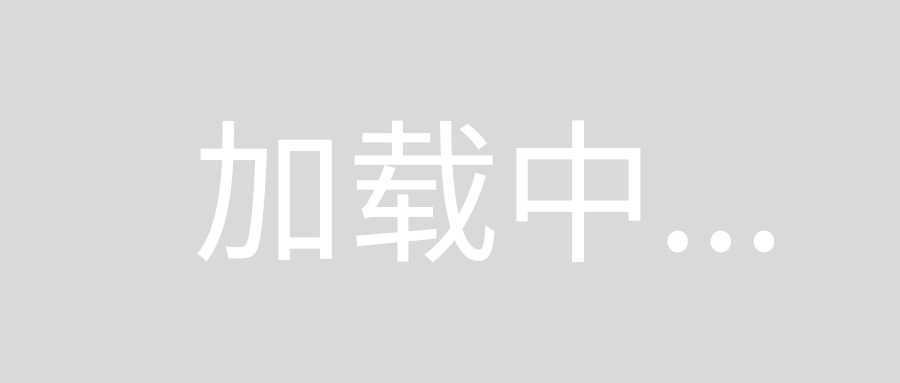
EDIT
An alternate pretty Ghetto solution using grid
tibble(x = as.factor(c('good', 'neutral', 'bad')),
y = as.factor(c('bad', 'neutral', 'bad'))) %>%
ggplot(aes(x = x, y = y)) +
geom_point()
grid::grid.polygon(x = c(.3,.3,.25,.25), y = c(.07,.04,.04,.07),gp = gpar(col = 'green', fill = 'green', alpha = .5))
grid::grid.polygon(x = c(.525,.525,.575,.575), y = c(.07,.04,.04,.07),gp = gpar(col = 'red', fill = 'red', alpha = .5))
grid::grid.polygon(x = c(.79,.79,.86,.86), y = c(.07,.04,.04,.07),gp = gpar(col = 'blue', fill = 'blue', alpha = .5))
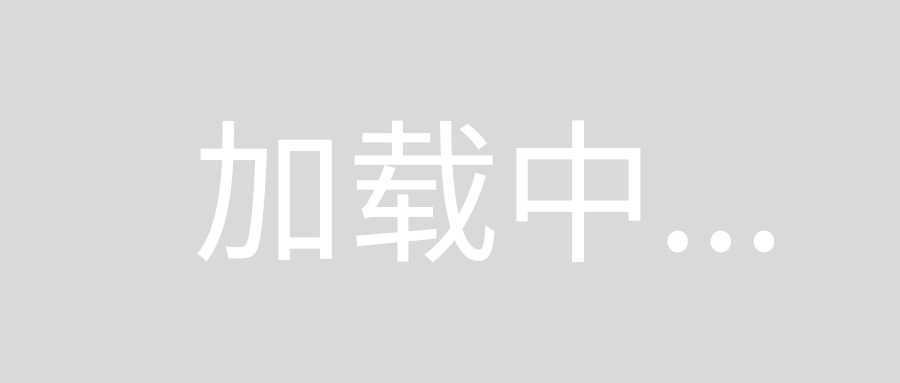
回答3:
Another approach
Create a vector of colors and pass them into axis.text.x()
option of theme()
.
# data
x = as.factor(c('good', 'neutral', 'bad'))
y = as.factor(c('bad', 'neutral', 'bad'))
df<- data.frame(x,y)
# create a vector of colors
mycolors<- c("red","blue","green")
library(ggplot2)
ggplot(df, aes(x = x, y=y))+
geom_point()+
theme(axis.text.x = element_text(colour = mycolors))
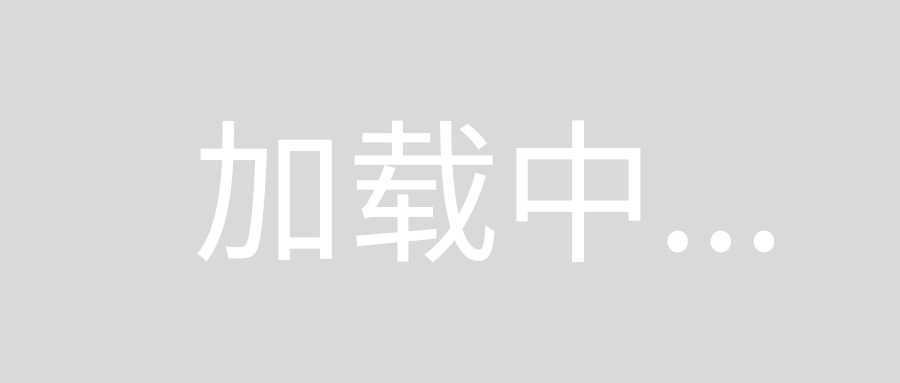
回答4:
One approach could be this:
tibble(x = as.factor(c('good', 'neutral', 'bad')),
y = as.factor(c('bad', 'neutral', 'bad'))) %>%
ggplot(aes(x = x, y = y)) + geom_point()+
geom_rect(aes(xmin=0.5, xmax=1.5, ymin=-Inf, ymax=Inf), fill="red", alpha=0.1)+
geom_rect(aes(xmin=1.5, xmax=2.5, ymin=-Inf, ymax=Inf), fill="yellow", alpha=0.1)+
geom_rect(aes(xmin=2.5, xmax=3.5, ymin=-Inf, ymax=Inf), fill="green", alpha=0.1)
With geom_rect()
you can add colored backgrounds:
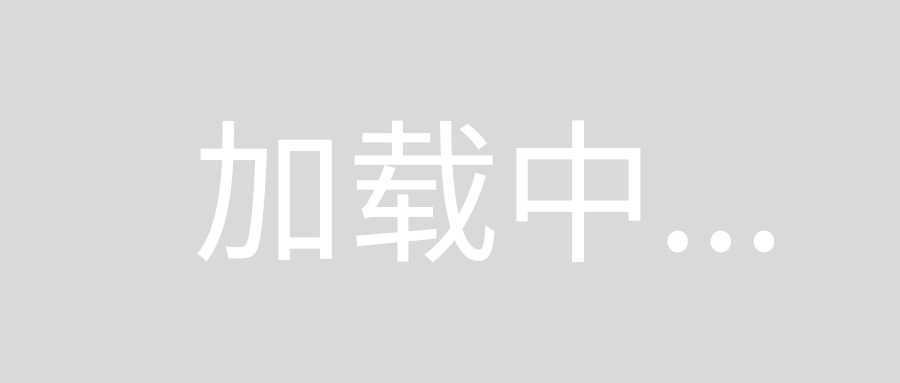