In the asp .net controller when defining an action, we can provide a name to the route as part of the [Route] attribute. In the below example, I've given the name as 'DeleteOrder'. How do I get to showing the name in the generated swagger documentation? Thanks.
[HttpDelete]
[Route("order/{orderId}", Name ="DeleteOrder")]
[ProducesResponseType(typeof(void), 204)]
[ProducesResponseType(typeof(void), 400)]
public async Task<IActionResult> Delete(string orderId)
By default, Swagger UI will list operations by their route. A non-intrusive way to include the route name into collapsed operations in Swagger UI would be to inject them in your operation's summary. Swashbuckle writes the summary field to the right of the HTTP Method and Route for each operation.
We can use an IOperationFilter to check each controller method for a Route Name and inject it into our summary. I've included a sample class AttachRouteNameFilter to start with:
using Swashbuckle.Swagger;
using System.Linq;
using System.Web.Http;
using System.Web.Http.Description;
namespace YourSpace
{
public class AttachRouteNameFilter : IOperationFilter
{
public void Apply(Operation operation,
SchemaRegistry schemaRegistry,
ApiDescription apiDescription)
{
string routeName = apiDescription
?.GetControllerAndActionAttributes<RouteAttribute>()
?.FirstOrDefault()
?.Name;
operation.summary = string.Join(" - ", new[] { routeName, operation.summary }
.Where(x => !string.IsNullOrWhiteSpace(x)));
}
}
}
Next, wire up this new Operation Filter in your Swagger configuration:
config.EnableSwagger(c =>
{
// Other configuration likely already here...
c.OperationFilter<AttachRouteNameFilter>();
});
Now start your app and observe that your route's name is visible before the operation's summary. Below is an example where my Route Name is 'GetMuffins':
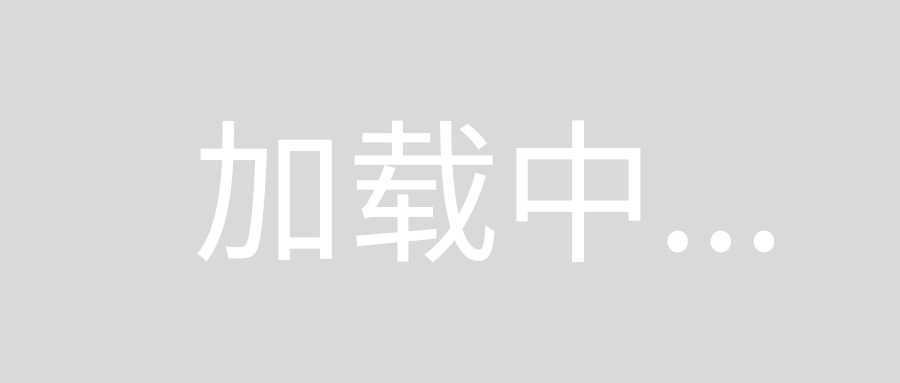
Further Reading
- Swashbuckle Documentation - Operation Filters
- Swashbuckle Documentation - Including XML Comments