I have to get the current country in the iPhone settings. Can anyone tell me how to get the current country in iPhone application.
I have to use the current country for parsing the RSS feed in which I need to pass the current country.
Please help me out to find the country .
Thanks in advance.
To find the country of the user's chosen language:
NSLocale *currentLocale = [NSLocale currentLocale]; // get the current locale.
NSString *countryCode = [currentLocale objectForKey:NSLocaleCountryCode];
// get country code, e.g. ES (Spain), FR (France), etc.
In Swift:
let currentLocale = NSLocale.currentLocale()
let countryCode = currentLocale.objectForKey(NSLocaleCountryCode) as? String
If you want to find the country code of the current timezone, see @chings228's answer.
If you want to find the country code of the device's physical location, you will need CoreLocation with reverse geocoding. See this question for detail: How can I get current location from user in iOS
NSLocale *locale = [NSLocale currentLocale];
NSString *countryCode = [locale objectForKey: NSLocaleCountryCode];
NSString *country = [locale displayNameForKey: NSLocaleCountryCode value: countryCode];
NSLocale *countryLocale = [NSLocale currentLocale];
NSString *countryCode = [countryLocale objectForKey:NSLocaleCountryCode];
NSString *country = [countryLocale displayNameForKey:NSLocaleCountryCode value:countryCode];
NSLog(@"Country Code:%@ Name:%@", countryCode, country);
//Country Code:IN Name:India
Source Link.
For devices with SIM card you can use this:
CTTelephonyNetworkInfo * network_Info = [CTTelephonyNetworkInfo new];
CTCarrier * carrier = network_Info.subscriberCellularProvider;
NSString * countryCode = [carrier.isoCountryCode uppercaseString];
If you're testing and want to use a different language from your system, it's easiest to change your scheme's Application Language
and Application Region
options instead of changing the language and region on your device or simulator.
Go to Product
->Scheme
->Edit Scheme...
->Run
->Options
and choose the Application Language
and Application Region
you want to test.
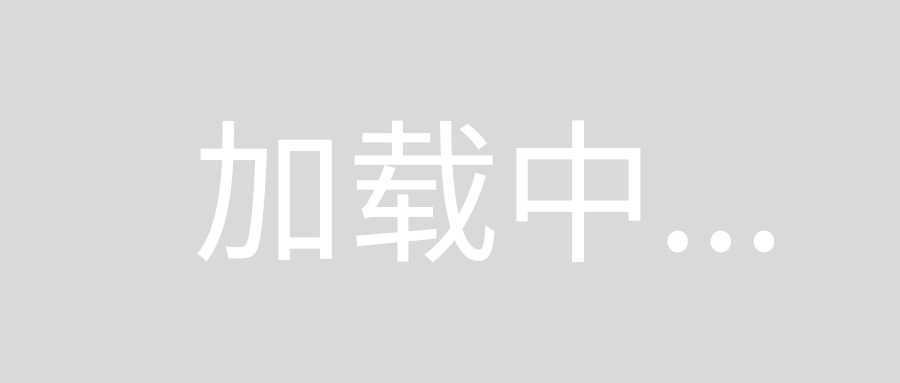
From the iOS Documentation: Testing Your Internationalized App
NSTimeZone *gmt = [NSTimeZone localTimeZone];
NSLog(@"timezone gmt %@",gmt.abbreviation);
Swift 3.0 solution
if let countryCode = (Locale.current as NSLocale).object(forKey: .countryCode) as? String {
print(countryCode)
}
Swift 3.0 latest working code is
if let countryCode = (Locale.current as NSLocale).object(forKey: .countryCode) as? String {
print(countryCode)
}
For Swift 4.0,
You can simply use
let countryCode = Locale.current.regionCode
print(countryCode)