I have a question regarding Google Charts (Column Charts) in specific.
"How do I make the "date" clickable so I can open up a modal dialog (jQuery) with an external page?"
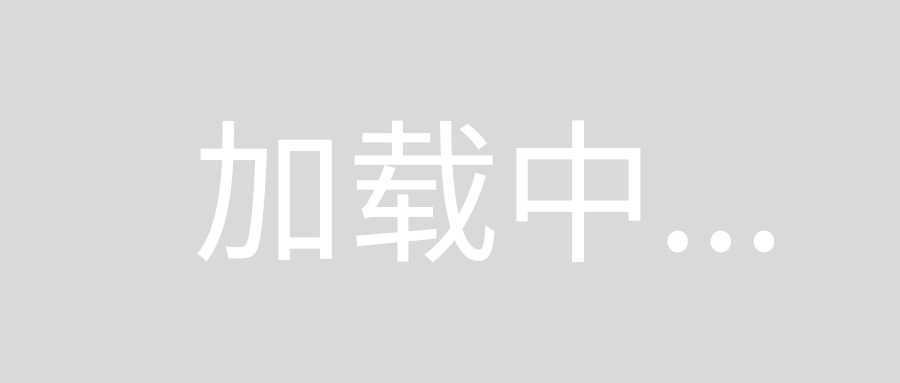
I have added an example to illustrate what I mean, this is obviously done in Photoshop.
I am able to bring up a alert dialog when I click any of the bars, but that's not really what I am looking for.
I have tried to search for it, but was unable to find something.
Attached is the code I used for making the bars clickable, maybe someone knows how to modify that without having to Google for it.
var handler = function(e) {
var sel = chart.getSelection();
sel = sel[0];
if (sel && sel['row'] && sel['column']) {
var message = "Hi..";
alert(message);
}
}
google.visualization.events.addListener(chart, 'select', handler);
Any assistance would be very much appreciated.
- Robert.
If you use a 'click'
handler instead of a 'select'
handler, you can more easily interact with other parts of the chart.
Here's an example: http://jsfiddle.net/6LJhv/6/
Your event object, e
, will have a targetID
property.
This targetID
is not well documented, but if you run the debugger, you can get a sense of what IDs look like.
On the various axis charts (Line, Column, etc.) the targetID
of the axis labels look something like this: hAxis#0#label#1
. To break that down, it means that you clicked on the second label of the first horizontal axis (0 based index).
Given that, we can dissect the targetID
to figure out which label you clicked on from the data if it's discrete.
(If your data is continuous, there isn't necessarily a 1:1 relationship with the labels and rows of data)
var handler = function(e) {
var parts = e.targetID.split('#');
if (parts.indexOf('label') >= 0) {
var idx = parts[parts.indexOf('label') + 1];
alert(data.getValue(0, parseInt(idx)));
}
};
google.visualization.events.addListener(chart, 'click', handler);