I'm trying to position my UIView to be 20pt under the navigation bar, but when I set it relative to the view on the view controller it's still under the navigation bar at 20pt, and I don't want to hardcode it.
Is it possible to position it away from the navigation bar?
Try adding contraint with TopLayoutGuide,
The main difference between TopLayoutGuide and self.view is, top layout guide starts with bottom of status bar + bottom of navigation bar(if exist)
, but self.view always start from (0,0) coordinate in iOS 7 for translucent navigation bar.
So in your case you should try pinning from top layout guide.
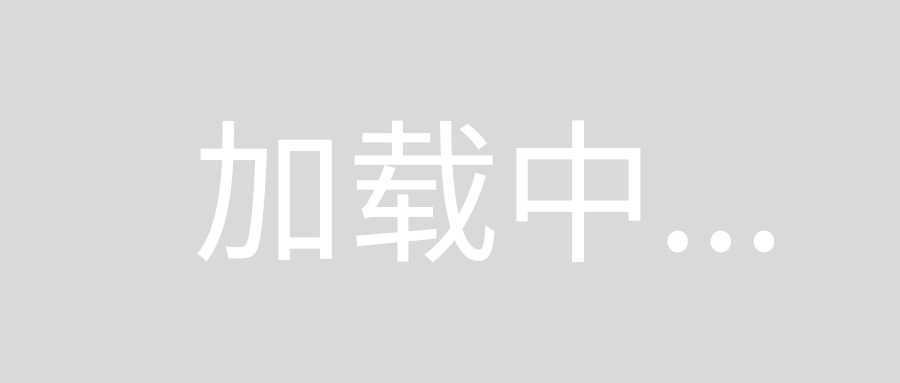
To do this programmatically use the topLayoutGuide
of your view controller:
override func viewDidLoad() {
...
myView.topAnchor.constraint(equalTo: self.topLayoutGuide.bottomAnchor).isActive=true
...
}
So your UIView sits within a UIViewController, correct?
When you define your initial inner UIView to that UIViewController, can you set its coordinates to be 20px off the top of the ViewController?
For example:
//
// ViewControllerEx.swift
//
import UIKit
class ViewControllerExample: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
var innerContainer = UIView(frame: CGRectMake(0, 20, self.view.bounds.width, self.view.bounds.height-20))
self.view.addSubview(innerContainer)
}
}