I have this code right here, and the idea is to have two buttons in a main window alongside a text area, which I have not added yet. After trying to use GridBagLayout and ripping off my hair in the process I decided not to use a layout and manually position buttons inside a non resizable window.
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
public class Tema extends JFrame implements ActionListener {
JMenuBar menubar = new JMenuBar();
JMenu actiuni = new JMenu("Actiuni");
JMenu contact = new JMenu("Contact");
JMenu help = new JMenu("Help");
JMenuItem ntest = new JMenuItem("Nou test");
JMenuItem vizarh = new JMenuItem("Vizualizare arhiva");
JMenuItem datcon = new JMenuItem("Date de contact");
JMenuItem sendmail = new JMenuItem("Trimite e-mail");
JMenuItem instrut = new JMenuItem("Instructiuni de utilizare");
JButton b1 = new JButton("Incepe testul!");
JButton b2 = new JButton("Vezi arhiva!");
JTextArea ta = new JTextArea("Default text", 5, 30);
public void common(String s)
{
setSize(800,450);
setLocationRelativeTo(null);
setResizable(false);
setTitle(s);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
menubar.add(actiuni);
menubar.add(contact);
menubar.add(help);
actiuni.add(ntest);
actiuni.add(vizarh);
contact.add(datcon);
contact.add(sendmail);
help.add(instrut);
setJMenuBar(menubar);
}
public Tema()
{
common("Self-Esteem- Fereastra Principala");
JPanel cp = new JPanel();
cp.setLayout(null);
b1.setBounds(100,100,200,100);
cp.add(b1);
b2.setBounds(100,250,200,100);
cp.add(b2);
setContentPane(cp);
setVisible(true);
}
public static void main(String[] args)
{
Tema x = new Tema();
}
@Override
public void actionPerformed (ActionEvent e){
}
}
But the output is this:
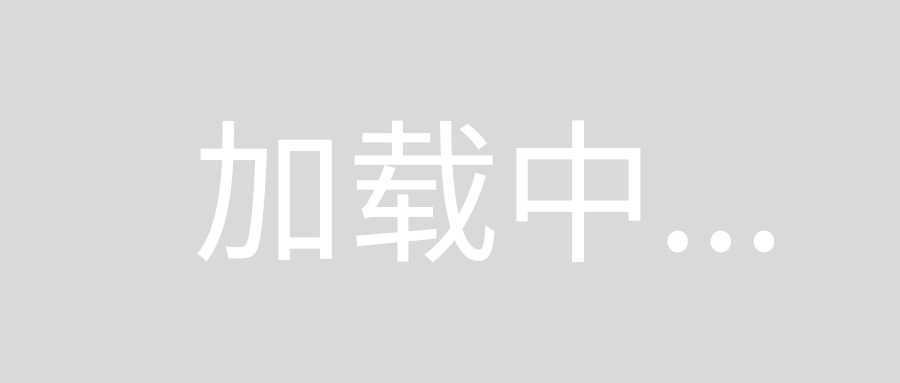
My question is why isn't the space beneath the second button equal to the space above the first button? Shouldn't they both be 100 pixels?
- Don't extend
JFrame
class unnecessarily.
- Never use
Absolute
/Null
LayoutManager
. Use an appropriate LayoutManager
, this includes nesting Layouts to achieve desired look. see here for good tutorials:
- A Visual Guide to Layout Managers
- Using Layout Managers
- Don't call
JFrame#setSize(..)
on JFrame
rather just call JFrame#pack()
before setting JFrame
visible.
- Dont use
JFrame#setContentPane(...)
just use add(..)
on JFrame
instance
- Create Event Dispatch Thread to initialize and change UI components
- Don't implement single
ActionListener
for multiple components. Unless it will be accessed by other class(es) or components share a Action
. Rather use an Anonymous ActionListener
Here is an example I made (basically your code fixed) hope it helps:
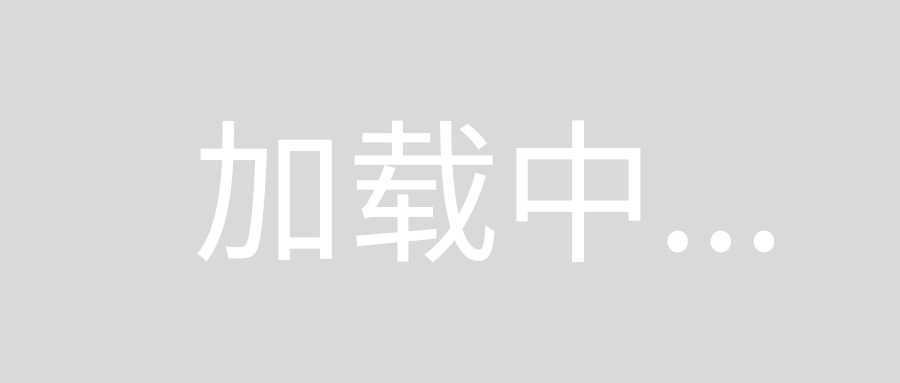
import java.awt.BorderLayout;
import java.awt.GridLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
import javax.swing.JTextArea;
import javax.swing.SwingUtilities;
public class LayoutTest {
private final JMenuBar menubar = new JMenuBar();
private final JMenu actiuni = new JMenu("Actiuni");
private final JMenu contact = new JMenu("Contact");
private final JMenu help = new JMenu("Help");
private final JMenuItem ntest = new JMenuItem("Nou test");
private final JMenuItem vizarh = new JMenuItem("Vizualizare arhiva");
private final JMenuItem datcon = new JMenuItem("Date de contact");
private final JMenuItem sendmail = new JMenuItem("Trimite e-mail");
private final JMenuItem instrut = new JMenuItem("Instructiuni de utilizare");
private final JButton b1 = new JButton("Incepe testul!");
private final JButton b2 = new JButton("Vezi arhiva!");
private final JTextArea ta = new JTextArea("Default text", 5, 30);
//create JFrame instance
private final JFrame frame = new JFrame();
public LayoutTest() {
initComponents();
}
public static void main(String[] args) {
//creat UI on EDT
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new LayoutTest();
}
});
}
private void initComponents() {
frame.setTitle("Self-Esteem- Fereastra Principala");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
menubar.add(actiuni);
menubar.add(contact);
menubar.add(help);
actiuni.add(ntest);
actiuni.add(vizarh);
contact.add(datcon);
contact.add(sendmail);
help.add(instrut);
frame.setJMenuBar(menubar);
JPanel textAreaJPanel = new JPanel();
//create button panel with GridLayout(2,1)
JPanel buttonJPanel = new JPanel(new GridLayout(2, 1));//new GridLayout(2, 1,10,10) creates gridlayout with horixontal and vertcial spacing of 10
//add buttons to one panel
buttonJPanel.add(b1);
buttonJPanel.add(b2);
//add text area to textarea jPanel
textAreaJPanel.add(ta);
//add textarea panel to west of content pane (BorderLayout by default)
frame.add(textAreaJPanel, BorderLayout.WEST);
//add button Panel to EAST of JFrame content pane
frame.add(buttonJPanel, BorderLayout.EAST);
frame.pack();
frame.setResizable(false);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
In the above code space between both the buttons is 50px, if you use b2.setBounds(100,300,200,100); then the space will be 100px. Try it...