I'm doing webscraping to this web:
http://www.falabella.com.pe/falabella-pe/category/cat40536/Climatizacion?navAction=push
I just need the information from the products: "brand", "name of product", "price".
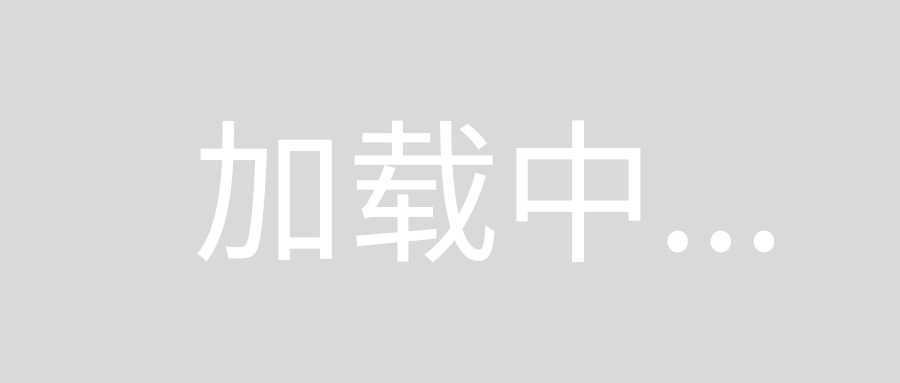
I can get that, but also i get the information from a banner with similar products by other users. I don't need it.
But when i go to the source code of the page, i can't see those products. I think it's been pulled through javascript or something:
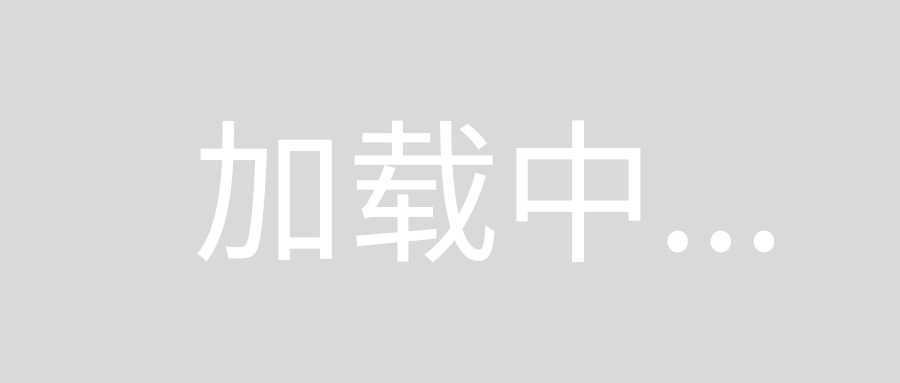
QUESTION 1: How to block this information when doing the web scraping?
This adds products that i don't need. But can't see this part in the source code.
QUESTION 2: When extracting prices "precio1", i get this as first element: "\n\t\t\t\tSubtotal InternetS/. 0"
I can't see that in the code source neither. How to not scrape it?
library(RSelenium)
library(rvest)
#start RSelenium
checkForServer()
startServer()
remDr <- remoteDriver()
remDr$open()
#navigate to your page
remDr$navigate("http://www.falabella.com.pe/falabella-pe/category/cat40536/Climatizacion?navAction=push")
page_source<-remDr$getPageSource()
Climatizacion_marcas1 <- html(page_source[[1]])%>%
html_nodes(".marca") %>%
html_nodes("a") %>%
html_attr("title")
Climatizacion_producto1 <- html(page_source[[1]])%>%
html_nodes(".detalle") %>%
html_nodes("a") %>%
html_attr("title")
Climatizacion_precio1 <- html(page_source[[1]])%>%
html_nodes(".precio1") %>%
html_text()
Staying close to your approach, this will do it:
library(rvest)
u <- "http://www.falabella.com.pe/falabella-pe/category/cat40536/Climatizacion?navAction=push"
doc <- html(u)
Climatizacion_marcas1 <- doc %>%
html_nodes(".marca")[[1]] %>%
html_nodes("a") %>%
html_attr("title")
Climatizacion_producto1 <- doc %>%
html_nodes(".detalle") %>%
html_nodes("a") %>%
html_attr("title")
The "\n\t\t" etc. comes from the parsing of the html. Apparently, there are carriage returns and tabs in there. A simple solution is:
Climatizacion_precio1 <- doc %>%
html_node(".precio1") %>%
html_text() %>%
stringr::str_extract_all("[:number:]{1,4}[.][:number:]{1,2}", simplify = TRUE) %>%
as.numeric
Climatizacion_precio1
[1] 44.9
This, in fact, picks the number from the string (thus also removing the "S/.". In case you want the "S/." to stay, you can do the following:
Climatizacion_precio1 <- doc %>%
html_node(".precio1") %>%
html_text() %>%
gsub('[\r\n\t]', '', .)
Climatizacion_precio1
[1] "S/. 44.90"
EDIT
Here is an alternative approach, using XML
and selectr
. This will get the info for all of the items on the page in one go.
library(XML)
clean_up <- function(x) {
stringr::str_replace_all(x, "[\r\t\n]", "")
}
product <- selectr::querySelectorAll(doc, ".marca") %>%
xmlApply(xmlValue) %>% lapply(clean_up) %>% unlist
details <- selectr::querySelectorAll(doc, ".detalle a") %>%
xmlApply(xmlValue) %>%
unlist
price <- selectr::querySelectorAll(doc, ".precio1") %>%
xmlApply(xmlValue) %>% lapply(clean_up) %>% unlist
as.data.frame(cbind(product, details, price))
product details price
1 Imaco Termoventilador NF15... S/. 44.90
2 Imaco Ventilador de 10" I... S/. 69
3 Imaco Ventilador Imaco de ... S/. 89
4 Taurus Recirculador TRA-30 ... S/. 89
5 Imaco Termoventilador ITC-... S/. 109
6 Sole Termo Ventilador Elé... S/. 99
7 Taurus Ventilador TVP-40 3 ... S/. 99
8 Imaco Estufa OFR7AO 1.500 ... S/. 129
9 Alfano Ventilador Recircula... S/. 139
10 Taurus Ventilador TVC-40RC ... S/. 139
11 Imaco Ventilador Pedestal ... S/. 149
12 Alfano Ventilador Orbital 1... S/. 149
13 Electrolux Ventilador de Mesa ... S/. 149.90
14 Alfano Estufa Termoradiador... S/. 159
15 Alfano Ventilador Pared 18"... S/. 169
16 Imaco Termoradiador OFR9AO S/. 179
You would normally probably want to do some initial cleaning of the results.