I'm getting the following error when trying to import a stored proc as a function in Entity Framework 5. I have recently updated the data project to reference the new version of EF.
The type parameter 'SSDS.Data.testy_Result' in ExecuteFunction is
incompatible with the type 'SSDS.Data.testy_Result' returned by the
function.
I can't get it to work for any stored proc...here is my simple test one:
CREATE PROCEDURE testy
AS
BEGIN
select 'hello' as hello
END
GO
It breaks with the exception above here:
public virtual ObjectResult<testy_Result> testy()
{
return ((IObjectContextAdapter)this).ObjectContext.ExecuteFunction<testy_Result>("testy");
}
And there is no error when I set the result to a string scalar in the Edit Function Import window in the model designer.
I'm calling the function like this:
private Entities db = new Entities();
var x = db.testy();
Is there something obvious that I'm missing here? There are a few edmx files in my project and the others were created with an older version of EF (and use ObjectContext).
Function Mappings:
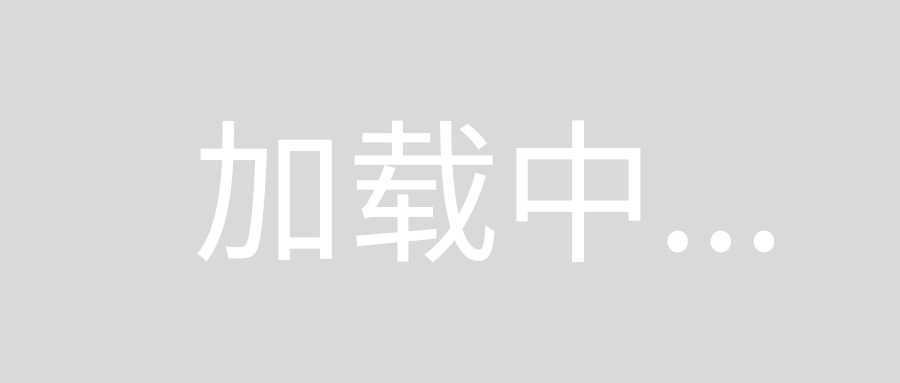
More Function Mappings Detail:
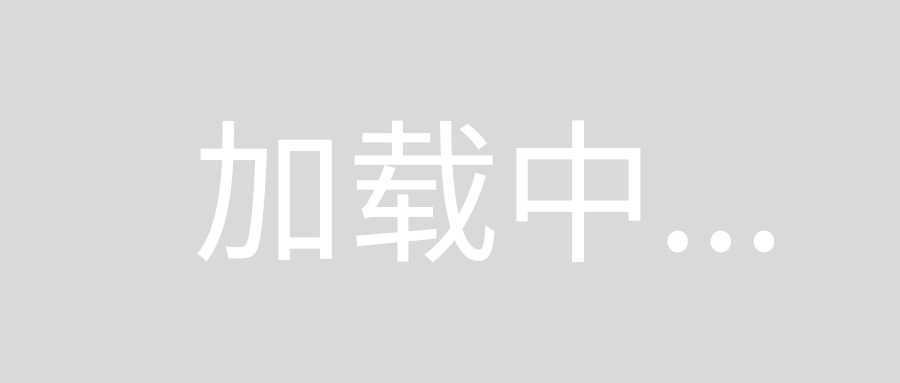
testy_Result class:
public partial class testy_Result
{
public string hello { get; set; }
}
I've been struggling with this same problem for the last few days. My project had also been upgraded from a previous version of Entity Framework to 5.0. I finally figured out it was due to having a mix of old and new edmxs. Removing the old edmx allowed the new one to work, but of course that's not a viable solution. I was also able to get it to work by changing the code generation strategy for the new (EF 5) edmx to "Legacy ObjectContext". If you're not familiar, this can be found by opening the edmx diagram, right-clicking, and going to Properties. I suspect all edmxs in the project just need to be on the same code generation strategy, so you could probably also change your old ones to T4 instead, although that would probably involve more work.
I do not know if this helps, but here is what I generally do:
Assuming that you have the following POCO class from the Entity Framework:
using System;
public partial class testy_Result
{
public string hello { get; set; }
}
The following call generally does the trick for me:
public partial class Entities: DbContext
{
public virtual ObjectResult<testy_Result> testy()
{
((IObjectContextAdapter)this).ObjectContext.MetadataWorkspace.LoadFromAssembly(typeof(testy_Result).Assembly);
return ((IObjectContextAdapter)this).ObjectContext.ExecuteFunction<testy_Result>
("testy", MergeOption.OverwriteChanges);
}
}
Also, just in case, I wrap my calls inside the using clause:
try
{
using (Models.Data.Entities Entities = new Models.Data.Entities())
{
var x = Entities.testy().FirstOrDefault().hello;
}
}
catch (Exception ex)
{
System.Diagnostics.Debug.WriteLine("Error: " + ex.Message);
}
Let me know if it worked for you.
Cheers.