My program reads in values from a file and uses a recursive method to print patterns of asterisks based on those values. I'm just having a problem getting everything to line up properly.
The output is supposed to look like this:
*
* *
* * *
* *
*
Regarding the format of the output, the directions are:
"Note that the pattern is aligned symmetrically (vertically) about the center line. The pattern should be aligned symmetrically on each line (horizontally) as well- hint: use the line value to help space."
But my output looks like this:
*
* *
* * *
* *
*
The code I'm using to get this pattern:
public static void makePattern(int thisRow, int num) {
if(thisRow >= num) {
for(int i = 0; i < num; i++) {
System.out.print(" " + "*" + " ");
}
System.out.println();
}
else {
for(int i = 0; i < thisRow; i++) {
System.out.print(" " + "*" + " ");
}
System.out.println();
makePattern(thisRow + 1, num);
for(int i = 0; i < thisRow; i++) {
System.out.print(" " + "*" + " ");
}
System.out.println();
}
}
Also my main method:
import java.util.Scanner;
import java.io.*;
public class Program3 {
public static void main(String[] args) throws Exception {
int num = 0;
int thisRow = 1;
java.io.File file = new java.io.File("../instr/prog3.dat");
Scanner fin = new Scanner(file);
while(fin.hasNext()) {
num = fin.nextInt();
if(num >=0 && num <= 25)
makePattern(thisRow, num);
System.out.println();
}
fin.close();
}
Any suggestions on how to edit my code to make my output appear like the example pattern I included?
Let's analyse the output first!!
First step is to analyse the output
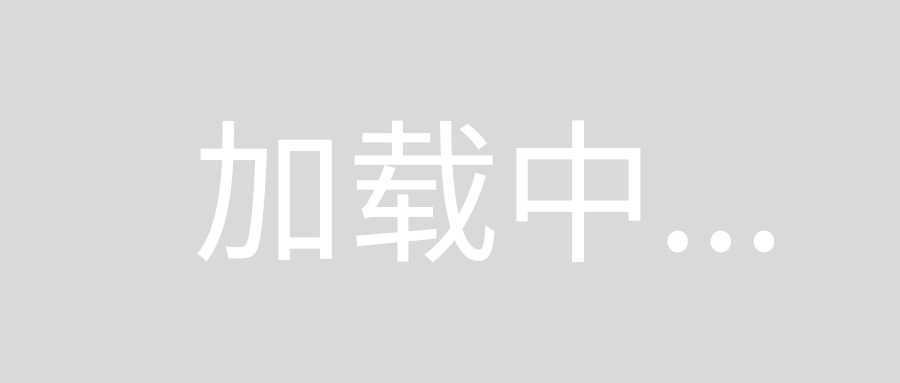
Conclusions:
- The total number of characters on every line is always n (=3)
Number of Spaces has the following pattern:
1st line 3 - 1 spaces
2nd line 3 - 2 spaces
3rd line 3 - 3 spaces
4th line 4 - 3 spaces
5th line 5 - 3 spaces
So
if(num < thisRow) {
numberOfSpaces = thisRow - num;
} else {
numberOfSpaces = num - thisRow;
}
Number of Stars is always [n - the number of spaces]
So
int numberOfStars = num - numberOfSpaces;
And the recursion should end on the 6th line, i.e. when current line number is n*2
So the return condition in your recursive method should be
if(thisRow == num * 2)
return;
Final Code : Putting the peices together
When we put the peices together, we get:
public static void makePattern(int thisRow, int num) {
//the termination condition
if(thisRow == num * 2)
return;
//the number of spaces
int numberOfSpaces = 0;
if(num < thisRow) {
numberOfSpaces = thisRow - num;
} else {
numberOfSpaces = num - thisRow;
}
//the number of stars
int numberOfStars = num - numberOfSpaces;
//compose the string before printing it
StringBuffer outputBuffer = new StringBuffer(num);
for (int i = 0; i < numberOfSpaces; i++){
outputBuffer.append(" ");
}
for (int i = 0; i < numberOfStars; i++){
outputBuffer.append("* ");
}
//print the string
System.out.println(outputBuffer.toString());
//recursion
makePattern(thisRow + 1, num);
}
This is code for printing diamond shaped pattern using recursion technique.
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.*;
public class patternRecursion {
static int n,k;
public static void main(String[] args) throws IOException{
try(Scanner s = new Scanner(System.in)){
n=Integer.parseInt(reader.readLine());
k=n-1;
printPattern(n);
}
}
public static void printChar(int m,char c){
if(m==0) return;
try{
printChar(m-1,c);
System.out.print(c);
}catch(StackOverflowError s){return;}
}
public static void printPattern(int m){
if(m==0){
return ;
}else{
printChar(m-1,' ');
printChar(n-m,'#');
printChar(n-m+1,'#');
System.out.println();
printPattern(m-1);
printChar(m,' ');
printChar(k-m,'#');
printChar(k-m+1,'#');
System.out.println();
}
}
}