可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
Between line 2 and 3 is a hidden <div>
. I don't want that one to be taken from the odd/even css
rule.
What would be the best approach to get this to work? http://jsfiddle.net/k0wzoweh/
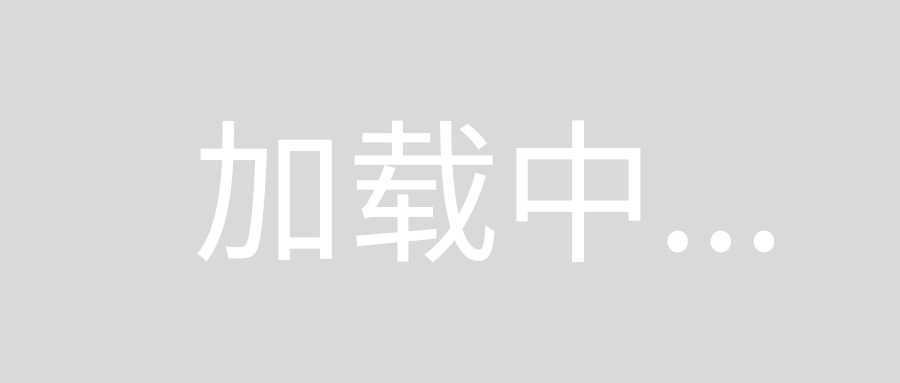
<style>
.box:not(.hidden):nth-child(even) {background: green}
.box:not(.hidden):nth-child(odd) {background: orange}
.hidden {display:none;}
</style>
<div class="wrap">
<div class="box">xx</div>
<div class="box">xx</div>
<div class="box hidden">xx</div>
<div class="box">xx</div>
<div class="box">xx</div>
<div class="box">xx</div>
<div class="box">xx</div>
</div>
Note: There can be multiple hidden
elements.
回答1:
Pseudo-selectors don't stack, so your :not
doesn't affect the :nth-child
(nor would it affect :nth-of-type
etc.
If you can resort to jQuery, you can use the :visible
pseudo-selector there, although that's not a part of the CSS spec.
If you're generating the HTML and can change that, you can apply odd/even with logic at run-time, eg in PHP:
foreach ($divs AS $i => $div) {
echo '<div class="box ' . ($i % 2 ? 'even' : 'odd') . '">x</div>';
}
Even trying to do something tricky like
.box[class='box']:nth-of-type(even)
doesn't work, because the psuedo-selector doesn't even stack onto the attribute selector.
I'm not sure there's any way to do this purely with CSS - I can't think of any right now.
回答2:
:nth-child()
pseudo-class looks through the children tree of the parent to match the valid child (odd
, even
, etc), therefore when you combine it with :not(.hidden)
it won't filter the elements properly.
Alternatively, we could fake the effect by CSS gradient as follows:
.hidden {display:none;}
.wrap {
line-height: 1.2em;
background-color: orange;
background-image: linear-gradient(transparent 50%, green 50%);
background-size: 100% 2.4em;
}
<div class="wrap">
<div class="box">xx</div>
<div class="box">xx</div>
<div class="box hidden">xx</div>
<div class="box">xx</div>
<div class="box">xx</div>
<div class="box">xx</div>
<div class="box">xx</div>
</div>
回答3:
Since my rows are being hidden with js, I found that the easiest approach for me was to just add an additional hidden row after each real row that I hide, and remove the hidden rows when I show the real rows again.
回答4:
Here's a CSS-only solution:
.box {
background: orange;
}
.box:nth-child(even) {
background: green;
}
.box.hidden {
display: none;
}
.box.hidden ~ .box:nth-child(odd) {
background: green;
}
.box.hidden ~ .box:nth-child(even) {
background: orange;
}
<div class="wrap">
<div class="box">xx</div>
<div class="box">xx</div>
<div class="box hidden">xx</div>
<div class="box">xx</div>
<div class="box">xx</div>
<div class="box">xx</div>
<div class="box">xx</div>
</div>
回答5:
Thanks Joe (+1) for pointing out the css pseudo-selector rules and the php code which I could pretty much use 1:1 in jQuery looking like this:
var classToAdd = visibleBoxes%2 ? 'even' : 'odd' ;
$(this).addClass(classToAdd)
回答6:
Hide the rows you want to hide calling .hide() for each table row, then call
$("tr:visible:even").css( "background-color", "" ); // clear attribute for all rows
$("tr:visible:even").css( "background-color", "#ddddff" ); // set attribute for even rows
(add your table name to the selector to be more specific)
(using :even makes it skip the Header row)
回答7:
As @Fateh Khalsa pointed out, I had a similar problem and since I was manipulating my table with JavaScript (jQuery to be precise), I was able to do the following:
(Note: This assumes use of JavaScript/jQuery which the OP did not state whether or not would be available to them. This answer assumes yes, it would be, and that we may want to toggle visibility of hidden rows at some point.)
- Inactive records (identified with the CSS class "hideme") are currently visible.
- Visitor clicks link to hide inactive records from the list.
- jQuery adds "hidden" CSS class to "hideme" records.
- jQuery adds additional empty row to the table immediately following the row we just hid, adding CSS classes "hidden" (so it doesn't show) and "skiprowcolor" so we can easily identify these extra rows.
This process is then reversed when the link is clicked again.
- Inactive records (identified with the CSS class "hideme") are currently hidden.
- Visitor clicks link to show inactive records from the list.
- jQuery removes "hidden" CSS class to "hideme" records.
- jQuery removes additional empty row to the table immediately following the row we just showed, identified by CSS class "skiprowcolor".
Here's the JavaScript (jQuery) to do this:
// Inactive Row Toggle
$('.toginactive').click(function(e) {
e.preventDefault();
if ($(this).hasClass('on')) {
$(this).removeClass('on'); // Track that we're no longer hiding rows
$('.wrap tr.hideme').removeClass('hidden'); // Remove hidden class from inactive rows
$('.wrap tr.skiprowcolor').remove(); // Remove extra rows added to fix coloring
} else {
$(this).addClass('on'); // Track that we're hiding rows
$('.wrap tr.hideme').addClass('hidden'); // Add hidden class from inactive rows
$('.wrap tr.hideme').after('<tr class="hidden skiprowcolor"></tr>');
// Add extra row after each hidden row to fix coloring
}
});
The HTML link is simple
<a href="#" class="toginactive">Hide/Show Hidden Rows</a>