We know that Xcode maintains environment variable of ${TARGET_NAME}
but how to access this variable in objective-C code ?
What I have tried ?
I have added "TARGET_NAME=${TARGET_NAME}"
this in Preprocessor macros section of Build Settings. But now I am not sure how to use this variable "TARGET_NAME"
as a string in objective-C code.
In my case product name and target name are different so, no chance to use that.
I tried to access using
#ifdef TARGET_NAME
NSLog(@"TargetIdentifier %@",TARGET_NAME);
#endif
This code is giving error like "Use of undeclared identifier 'myapptargetname'"
You can add "TargetName" key to your Info.plist file:
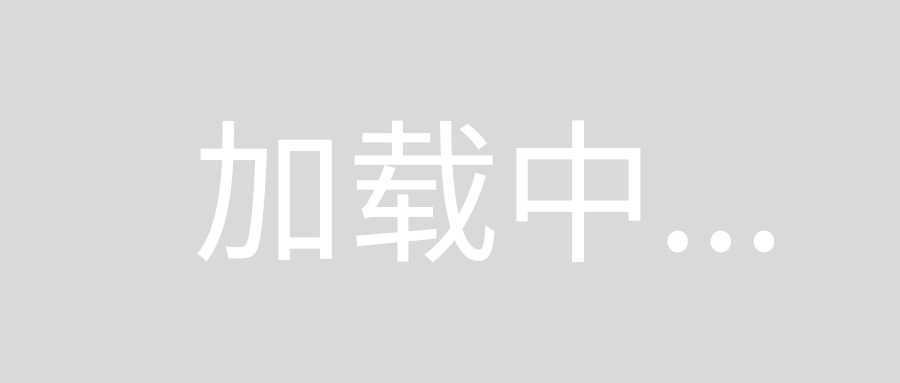
Then you can access it (swift code):
var plistFileName = NSBundle.mainBundle().infoDictionary?["TargetName"] as String
NSLog(@"Target name: %@",[[[NSBundle mainBundle] infoDictionary] objectForKey:@"CFBundleName"]);
Hope to help you!
Edited: "CFBundleName"
thanks Max and Daniel Bo for your commend
Swift 4, Xcode 9+
Bundle name:
Bundle.main.object(forInfoDictionaryKey: "CFBundleName") as? String ?? ""
Bundle display name:
Bundle.main.object(forInfoDictionaryKey: "CFBundleDisplayName") as? String ?? ""
Swift 3, Xcode 8+
let targetName = Bundle.main.infoDictionary?["CFBundleName"] as? String ?? ""
In Xcode 7.3.1
if let targetName = NSBundle.mainBundle().infoDictionary?["CFBundleName"] as? String{
print(targetName)
}
** For Swift 4 **
I am not sure how good it is to have a Constants file in Swift but you could create something like that:
enum TargetType:String, CaseIterable{
case target1 = "My Target 1"
case target 2 = "My Target 2"
case unknown
}
var currentTarget:TargetType = {
return TargetType(rawValue: (Bundle.main.object(forInfoDictionaryKey: "CFBundleName") as? String ?? "")) ?? .unknown
}()
So you can called it any time like that
if currentTarget != .unknown{
print(currentTarget.rawValue)
}
If you want to add the variable inside a Constants class then:
class Constants: NSObject {
static var currentTarget:TargetType = {
return TargetType(rawValue: (Bundle.main.object(forInfoDictionaryKey: "CFBundleName") as? String ?? "")) ?? .unknown
}()
}