Recently I started getting this issue, when I install a react-native package
(eg: react-navigation
) into my project, a whole bunch of packages are been removed (including react, react-native i think).
And then when i try to run command "run-android
", it says it doesn't recognize.
I recently updated to the latest npm
and react-native-cli
. Is it something wrong with "npm install
"? or react-native
?
node version: 8.1.2 <br/>
react-native-cli: 2.0.1 <br/>
react-native: 0.45.1 <br/>
react-navigation: 1.0.0-beta.11
Below are the steps to re-create:
Notice in the image above. Seems like all the other packages are removed from the project.<br/><br/>
- Step 4 - Try running "run-android" command again. (will fail, but used to work before)
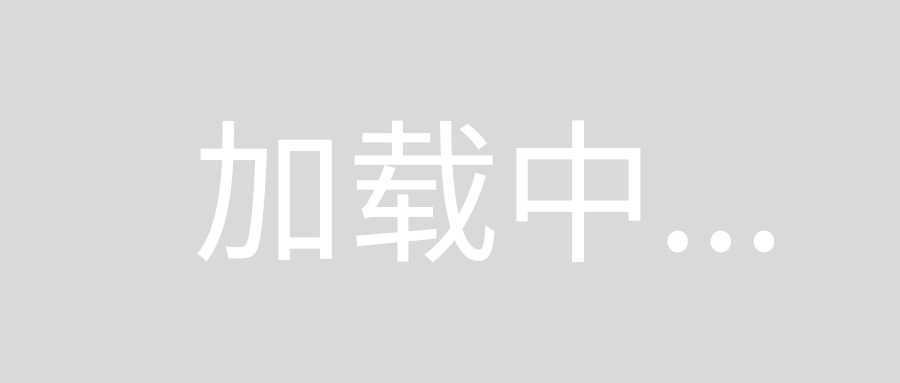
Any idea on what the issue is and any way to solve it?
Found the solution here.
At first running npm install
didn't work, but then, deleting the package-lock.json
file and running npm install
did the job.
After that I installed react-navigation
package seperately and it worked fine.
Here's what worked for me from start to finish.
react-native init NavTest
(The cli is locally installed with this command)
- deleted package-lock.json
npm install --save react-navigation
- deleted the generated package-lock.json
npm install
react-native run android
A little ugly, I don't know entirely what happened, but this worked.
https://reactnavigation.org/ for sample code to run.
Or Copy this to index.android.js
import React, { Component } from 'react';
import {
AppRegistry,
Button,
} from 'react-native';
import {
StackNavigator,
} from 'react-navigation';
class HomeScreen extends Component {
static navigationOptions = {
title: 'Welcome',
};
render() {
const { navigate } = this.props.navigation;
return (
<Button
title="Go to Jane's profile"
onPress={() =>
navigate('Profile', { name: 'Jane' })
}
/>
);
}
}
class ProfileScreen extends Component{
static navigationOptions = {
title: 'Jane',
};
render() {
return (null);
}
}
const NavTest= StackNavigator({
Home: { screen: HomeScreen },
Profile: { screen: ProfileScreen },
});
AppRegistry.registerComponent('NavTest', () => NavTest);