When I execute the code below in the simulator I expect to see red fill the screen, but instead it's all black, why?
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
UIView * myView = [[UIView alloc] initWithFrame:[window bounds]];
[myView setBackgroundColor:[UIColor redColor]];
[window addSubview:myView];
[window makeKeyAndVisible];
return YES;
}
If I add initialization of window, that doesn't help:
window = [[[UIWindow alloc] init] initWithFrame:[[UIScreen mainScreen] bounds]];
My troubles begun when after creating a Window-based Universal project in Xcode I decided to delete the iPad/iPhone xib files and the iPhone/iPad app delegate files that were automatically created in the project, and instead have a single app delegate with a view controller that builds the view programmatically based on the device. Now I can't seem to display a simple view I create in the app delegate.
Edit: I removed adding a view, and now set the background color of the window to red. That doesn't help, but if I go to desktop in the simulator and reopen the running app I get now a red screen. Again, I'm puzzled as to why I don't see red the first time I launch the app.
Here are the steps to setup a non-InterfaceBuilder (.xib) Universal iOS project.
Delete all associations to the .xib files in your property list for the application.
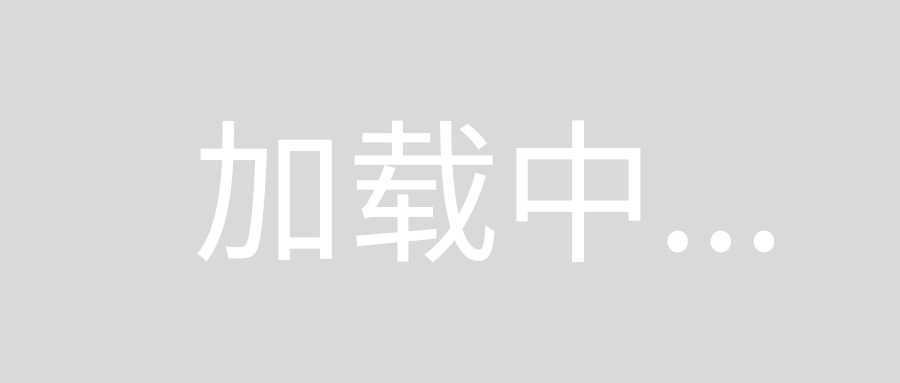
Delete all the .xib files from the project
- (Optional) Create a common Application Delegate class, and then delete the AppDelegate_iPad.* and the AppDelegate_iPhone.* files. (Copy paste any code from the existing files before deleting)
Change your main.m file to name the application delegate. I chose to modify and use the AppDelegate_iPhone for example purposes. See the documentation: UIApplication Reference
// main.m
int main(int argc, char *argv[]) {
NSAutoreleasePool * pool = [[NSAutoreleasePool alloc] init];
// Start an iPhone/iPad App using a .xib file (Defaults set in .plist)
//int retVal = UIApplicationMain(argc, argv, nil, nil);
// Start the iPhone/iPad App programmatically
// Set the 3rd argument to nil, to use the default UIApplication
// Set the 4th argument to the string name of your AppDelegate class
int retVal = UIApplicationMain(argc, argv, nil, @"AppDelegate_iPhone");
[pool release];
return retVal;
}
Update your application delegate code.
// AppDelegate_iPhone.m
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch.
NSLog(@"Launch my application iphone");
// Create the window, it's not created without a nib
window = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
// Create a subview that is red
UIView *myView = [[UIView alloc] initWithFrame:[window bounds]];
[myView setBackgroundColor:[UIColor redColor]];
// Add the subview and release the memory, sicne the window owns it now
[self.window addSubview:myView];
[myView release];
[self.window makeKeyAndVisible];
return YES;
}
I tried to run your code and it worked for me. I got en empty view that was coloured red. Have you tried adding breakpoints and checking that your code actually gets executed? Otherwise the only thing I can come up with is that it is something with your UIWindow.